File Logging in Windows Form Application using Serilog
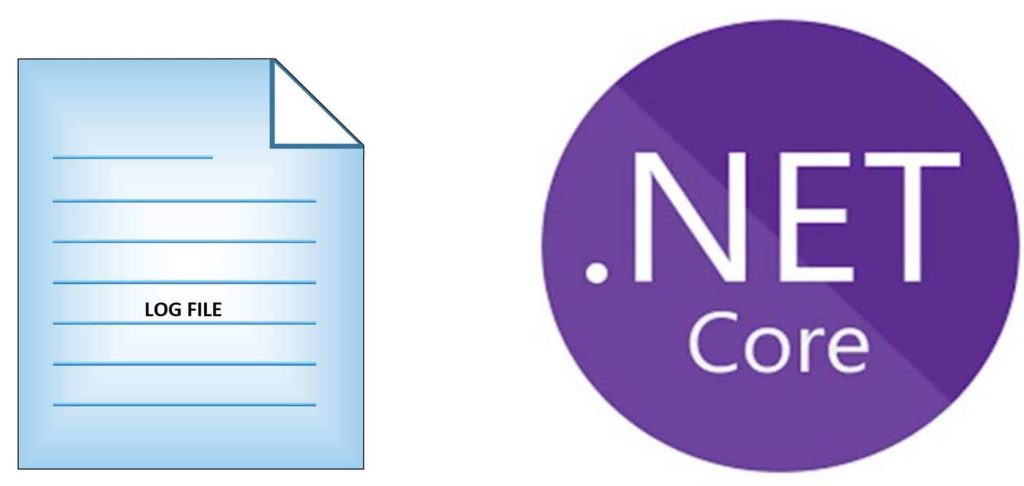
Today in this article, we will see how to achieve File Logging in Windows Form Applications using Serilog.
As we know .NET Core has introduced ILogger as a generic interface for logging purposes. This framework-supported interface ILogger can be used across different types of applications like ASP.NET API logging, Console App logging or Windows Form app logging using built-in providers.
Today in this article, we will cover below aspects,
We also discovered that the File/Rolling File-based logging provider is still not available through the .NET Core framework and we need to rely on external solutions.
Microsoft recommends using a third-party logger framework like a Serilog or NLog for other high-end logging requirements like Database or File/Rolling File logging.
Implement DI in Windows Forms
Unlike ASP.NET Core the Windows Forms app doesn’t have dependency injection built into the framework but uses a few approaches as discussed in the article Using Service provider for DI in Windows Forms App or using Generic HostBuilder for DI in Windows Forms App we can very much achieve the same.
We shall extend the same above-discussed sample for Serilog further.
However, the discussed approach is experimental and shows a possible approach of achieving logging in Windows forms.
Getting started
Here I am using a Forms/Windows .NET Core 3.1 application.

Please add below Serilog Nuget Packages
Serilog File Log
If you need basic File logging please add the below NuGet packages.
PM> Install-Package Serilog.Sinks.File -Version <version no>
OR
Serilog Rolling File Logging
Alternatively if for rolling file requirement please add below NuGet package.
PM> Install-Package Serilog.Sinks.RollingFile -Version 3.3.0
Additionally, please add below NuGet packages,
- Microsoft.Extensions.Hosting
- Serilog.Extensions.Logging
Please create Generic HosBuilder and register the dependencies that need to inject. These changes can be done in the Main() method.
I have added my business and data object below if you don’t have it, you can ignore those objects. Please add the Form object as Singleton or Scoped as per requirement.
var builder = new HostBuilder()
.ConfigureServices((hostContext, services) =>
{
services.AddScoped<Form1>();
services.AddScoped<IBusinessLayer, BusinessLayer>();
services.AddSingleton<IDataAccessLayer, CDataAccessLayer>();
});
Create Serilog Logger Configuration
Create Serilog Logger Configuration as shown below,
File Logging:
var serilogLogger = new LoggerConfiguration()
.WriteTo.File("TheCodeBuzz.txt")
.CreateLogger();
Rolling File Logging:
If using the Rolling File feature then use the configuration below,
var serilogLogger = new LoggerConfiguration()
.WriteTo.RollingFile("TheCodeBuzz.txt")
.CreateLogger();
Note: You should add the Nuget package 'Serilog.Sinks.RollingFile' to use this feature.
Here below is what the Main() methods look like as below.
The below example talks about the Dependency approach. However above object created i.e “serilogLogger” can be used in any inline code to add log as per need anywhere in the code without dependency injection.
[STAThread]
static async Task Main()
{
Application.SetHighDpiMode(HighDpiMode.SystemAware);
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
///Generate Host Builder and Register the Services for DI
var builder = new HostBuilder()
.ConfigureServices((hostContext, services) =>
{
services.AddScoped<Form1>();
services.AddScoped<IBusinessLayer, BusinessLayer>();
services.AddSingleton<IDataAccessLayer, CDataAccessLayer>();
//Add Serilog
var serilogLogger = new LoggerConfiguration()
.WriteTo.File("TheCodeBuzz.txt")
.CreateLogger();
services.AddLogging(x =>
{
x.SetMinimumLevel(LogLevel.Information);
x.AddSerilog(logger: serilogLogger, dispose: true);
});
});
var host = builder.Build();
using (var serviceScope = host.Services.CreateScope())
{
var services = serviceScope.ServiceProvider;
try
{
var form1 = services.GetRequiredService<Form1>();
Application.Run(form1);
Console.WriteLine("Success");
}
catch (Exception ex)
{
Console.WriteLine("Error Occured");
}
}
}
Implementation for class ‘Form1’ as below,
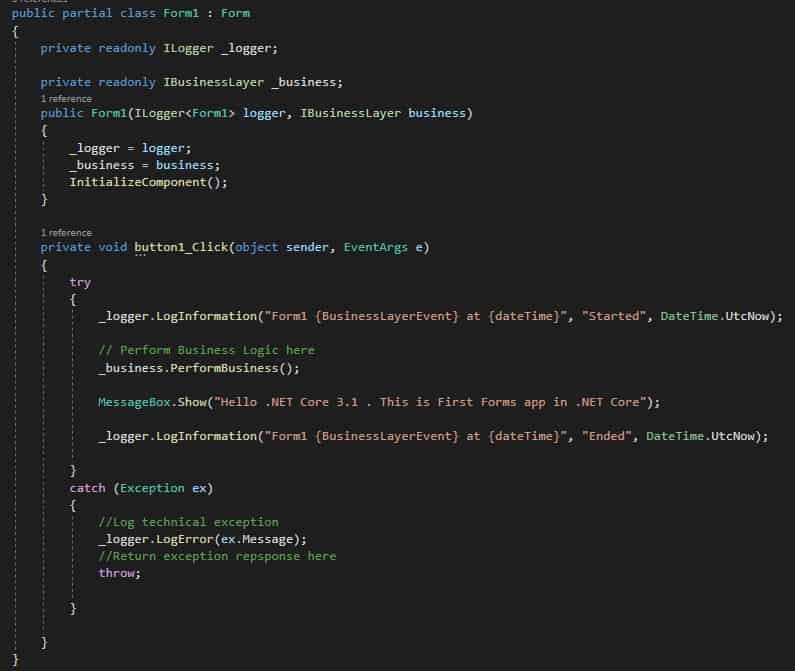
Let’s run the application and check the log details captured in a file.

Logfile shall be generated in the app root folder itself if the path is not specified. Please specify your custom file path and other configuration in WriteTo.File(..) or AddLogging() methods as required.
Summary
Serilog helps us enable logging in a few simple steps and addresses the file-logging requirement easily in .NET Core-based Desktop or Windforms applications. As a file logging provider is not yet available through the .NET Core framework and certainly, we can use Serilog for any File-based logging requirements easily.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
AddSerilog not found
Hello Kiquenet – I hope you added the required Nuget package and below using the namespace.
Microsoft.Extensions.Hosting
Serilog.Extensions.Logging
thx a lot, Serilog.Extensions.Logging is required.
finally working