Javascript Development Best Practices
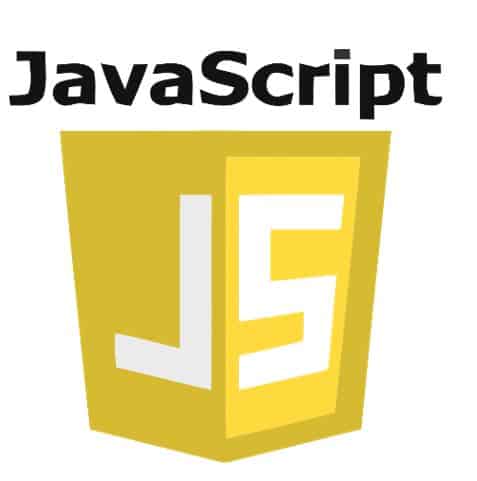
Coding Best practices and Guidelines are always helpful in the long run for any language used for application development.
These best practices improve our code quality, make our maintainable, bring uniformity, avoid ambiguity, and importantly help make you a good Developer.
The below guidelines are meant to be more for fresh and experienced Javascript learners.
Today, we shall see high-level Javascript guidelines to be used for any code development application.
Today in this article, we will cover below aspects,
Single Responsibility Function
You must modularize your application using single-responsibility functions. Single-responsibility functions are simple, small functions that do one task at a time.
- It means to build your functions as small as possible
- Small functions are easier to test and maintain.
- Small functions promote reading and reuse.
- Small functions help avoid hidden bugs
Use ES6 and the Above features
Leverage and use all new features brought in with ECMAScript 6 which helps you to develop responsive Web Design.
- let
- const
- Arrow Functions
- Classes
- Array.find()
- Array.findIndex()
Minimize/Avoid Global Variables
Global variables and functions can easily be overwritten by other scripts.
This is because the JavaScript file on the page runs in the same scope.
So when you have global variables in your code, scripts that contain the same variable and function use get overwritten.
Strict Coding Style
Browsers ignore coding issues when it comes to Bad syntax in your Javascript code.
It’s recommended to always use Strict mode while programming JavaScript. This behavior can be implemented by multiple measures like,
- Using the “use strict” keyword
- Using linting – like jslint, eslint, sonarlint etc.
Note- “use strict” should be used at the beginning of a script or a function to make it work.
Optimize the DOM access
Write the Javascript function with the optimized way of accessing DOM.
- DOM’s are complex API
- Accessing DOM multiple times in code could cause performance issues.
- Use other techniques like template-based elements( Angular 8,9) or Virtual DOM (React)
Code for Future
Write Javascript code with all possible new features leveraging the latest feature and at the same time consider backward compatibility of old features.
Try using new features as per best practices so that these keywords remain usable for any future coding.
Useful keywords,
- interface
- let
- private
- protected
- public
- static
- yield
Additionally, try following progressive enhancement and Graceful degradation principles to make your code future-proof.
Variable Declaration
Best practices on variable declaration are important in a lot of aspects which are explained below,
- Helps write clean code
- Provide a single location to define and declare local variables
- Avoid global variables declaration
- Avoid unused variables
- Avoid duplicate variable declaration
- Use strict declaration
Declare Local Variables
Always declare a local variable using the var and let keywords.
Always initialize Variables
As good practice initialize your variable same time when you declare it.
Javascript is not TypeSafe
Be aware while performing custom or explicit conversions.
JavaScript is loosely typed. A variable can contain different data types. One should be aware of any such conversion.
Sanitize the Data
Always Sanitize your UI inputs. Improper sanitization of inputs may lead to almost all of the major vulnerabilities in web applications, such as cross-site scripting, interpreter injection, locale/Unicode attacks, buffer overflow, etc.
“All Input is Evil” _ Michael Howard
Avoid heavy nesting
As discussed above It’s important you modularize your application using single-responsibility functions. Which ultimately helps you avoid heavy nesting logic.
If your nesting logic goes deeper consider refactoring the API making your API easy to read and maintain.
Browser Agnostic code
Remember technology change is inevitable but the best practices will help you keep running for the long run and adapt to the changes quickly.
- Write code following generic coding best practices
- Don’t target a particular browser only as things will change anytime
- Always manage browser-specific code separately and keep track of it
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.