Using Log4Net for Logging in Windows Form Application
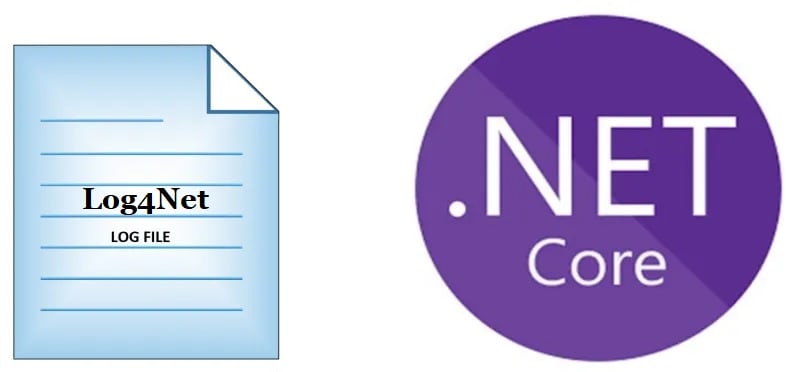
Today in this article, we will see how to use Log4Net for Logging in Windows Form Desktop Application .NET Core application.
As we understood .NET Core only provides basic providers for logging purposes like Console, EventSource, etc. File/Rolling File logging provider is still not available through the .NET Core framework.
However, we need to rely on external solutions and today we shall see how to use Log4Net to address the same requirement of File/Rolling File Logging or Console logging in the Windows Form/Desktop app.
Today in this article, we will cover below aspects,
We shall be leveraging the DI( Dependency Injection) framework to inject the Log4Net Logger object into the mini IoC DI container in a .NET Core Console application.
Microsoft recommends using a third-party logger framework like a Serlilog or Log4Net or NLog for high-end logging requirements like Database or File/Rolling File logging.
Using Log4NET, logging can be done on the Console or File or Rolling file easily.
Log4NET also provides flexibility to control log layout and log as per log level types in the application.
Getting Started
Here I am using a Forms/Windows .NET Core 3.1 application.
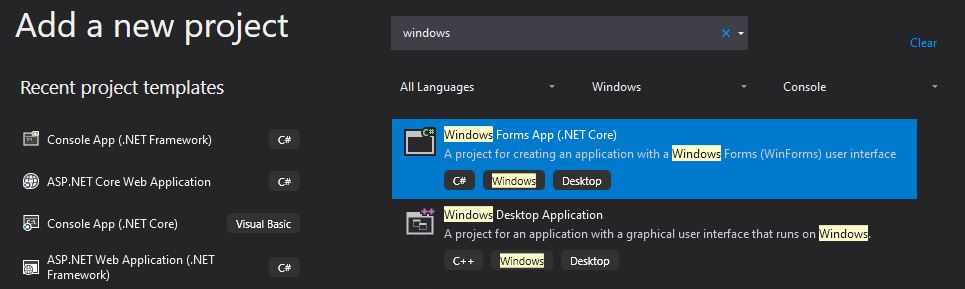
Creating Generic HostBuilder
We will be leveraging the DI( Dependency Injection) framework to inject the Log4Net Logger.
The HostBuilder class is available from the following namespace,
using Microsoft.Extensions.Hosting;
Please install the NuGet package from Nuget Package Manager or PMC,
PM> Install-Package Microsoft.Extensions.Hosting -Version 3.1.1
Configuring Log4Net
Log4Net is a Nuget package and is available through the NuGet packages manager.
PM> Install-Package Microsoft.Extensions.Logging.Log4Net.AspNetCore -Version 3.1.0
Or
Please install the package through Nuget Package Manager,
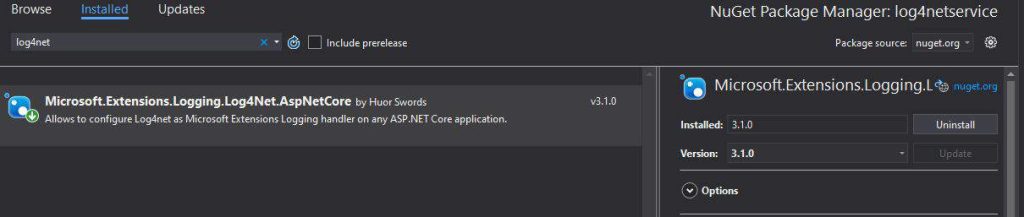
Please create Generic HosBuilder and register the dependencies like Log4Net and other business dependencies.
See below the implementation for HostBuilder and registration for Log4Net logging.
These changes can be done in the Main() method,
var builder = new HostBuilder()
.ConfigureServices((hostContext, services) =>
{
services.AddSingleton<Form1>();
})
The next step is to register Log4Net in the IoC container as below,
///Generate Host Builder and Register the Services for DI
var builder = new HostBuilder()
.ConfigureServices((hostContext, services) =>
{
//Register all your services here
services.AddScoped<Form1>();
}).ConfigureLogging(logBuilder =>
{
logBuilder.SetMinimumLevel(LogLevel.Trace);
logBuilder.AddLog4Net("log4net.config");
});
Please add a new Log4Net.config file if not available with log layout details and configuration as given below as an example.
Below is the complete implementation for IoC container implementation which you need to add it to Main() method,
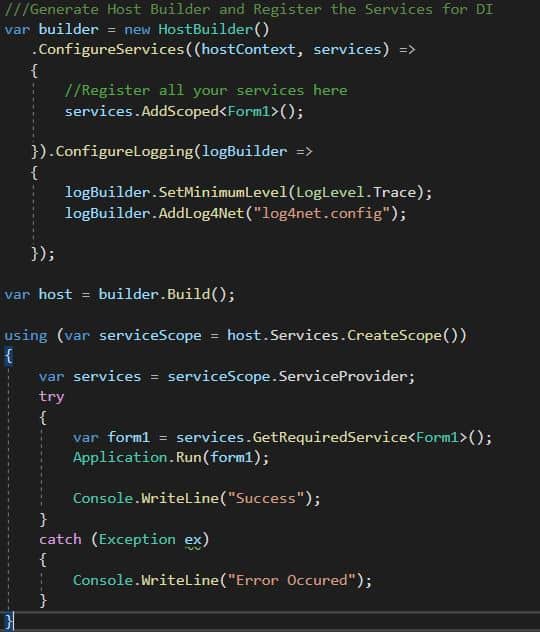
All set, Lets now define and do dependency injection of ILogger instance within Form Class as below,
public partial class Form1 : Form
{
private readonly ILogger _logger;
public Form1(ILogger<Form1> logger)
{
_logger = logger;
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
try
{
_logger.LogInformation("Form1 {BusinessLayerEvent} at {dateTime}", "Started", DateTime.UtcNow);
// Perform Business Logic here
MessageBox.Show("Hello .NET Core 3.1 . This is Log4NET logging in .NET Core Win Form");
_logger.LogInformation("Form1 {BusinessLayerEvent} at {dateTime}", "Ended", DateTime.UtcNow);
}
catch (Exception ex)
{
//Log technical exception
_logger.LogError(ex.Message);
//Return exception repsponse here
}
}
}
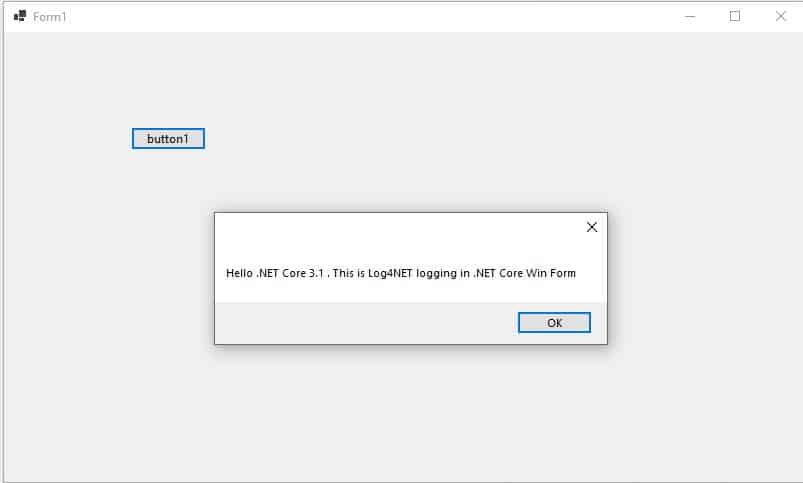
The log file shall be created in the project output directory unless specified with a custom path and logging will be captured as shown below figure.
Below is an example of file logging created by Log4Net,
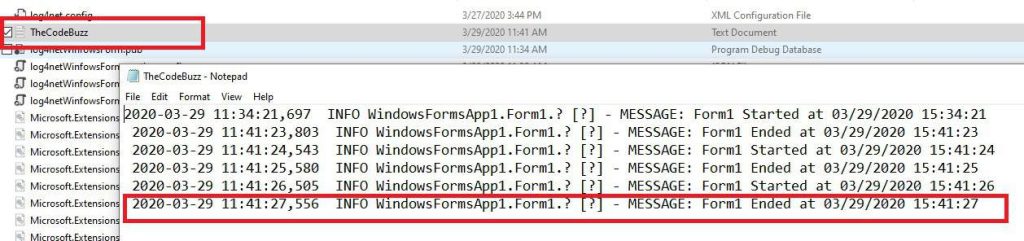
File Logging using File Appender
If you wish to enable only the File/Rolling File logging appender, please use the File appender in the log4net.config file as below,
Sample log4net.config file used is as below,
<log4net>
<appender name="RollingFile" type="log4net.Appender.RollingFileAppender">
<file value="TheCodeBuzz.log" />
<appendToFile value="true" />
<maximumFileSize value="100KB" />
<maxSizeRollBackups value="2" />
<layout type="log4net.Layout.PatternLayout">
<conversionPattern value="%date %5level %logger.%method [%line] - MESSAGE: %message%newline %exception" />
</layout>
</appender>
<root>
<level value="TRACE" />
<appender-ref ref="RollingFile" />
</root>
</log4net>
Logging using Console Appender
Below is sample log4net.config for the Console appender as below,
<appender name="Console" type="log4net.Appender.ConsoleAppender">
<layout type="log4net.Layout.PatternLayout">
<!-- Pattern to output the caller's file name and line number -->
<conversionPattern value="%date %5level %logger.%method [%line] - MESSAGE: %message%newline %exception" />
</layout>
</appender>
<appender-ref ref="ConsoleAppender" />
</root>
Log4NET also supports multiple appenders configured at a time.
References: Dependency Injection in Windows Form .NET Core
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Happy Coding !!
Summary
Today in this article we saw how to log in a .NET Core Windows Form application. Logging in Windows Form is simplified further. Log4Net helps us enable logging in a few simple steps and addresses the file-based and other types of logging requirements easily.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.