How to add Logging in Middleware using LoggerFactory
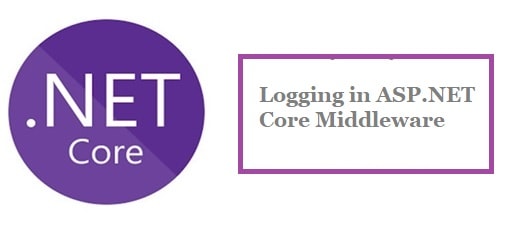
In this article, we will see how to perform Logging in Middleware using LoggerFactory in the ASP.NET Core application.
As we know Middleware is a component that is used in the application pipeline to handle requests and responses which can also help perform pre and post-operation within the API pipeline.
Today in this article, we will cover below aspects,
We shall use the approach of the middleware component for handling ILoggerFactory within the ASP.NET Core API pipeline.
Getting Started
Let’s create an ASP.NET Core 3.1 or .NET 5 application.
Let’s create a very basic middleware component as below,
Create Middleware component
Let’s create CustomMiddleware class as below,
public class CustomMiddleware
{
private readonly RequestDelegate _next;
private readonly ILoggerFactory _loggerFactory;
public CustomMiddleware(RequestDelegate next, ILoggerFactory loggerFactory)
{
_next = next;
_loggerFactory = loggerFactory;
}
I am here injecting RequestDelegate and ILoggerFactory from the constructor of CustomMiddleware.
Lets now add InvokeAsync(Assuming we need asynchronous middleware behavior) method as below,
public async Task InvokeAsync(HttpContext context)
{
var _logger = _loggerFactory.CreateLogger<CustomMiddleware>();
try
{
_logger.LogInformation("Performing Middleware operation");
// Perform some Database action into Middleware
_logger.LogWarning("Performing Middleware Save operation");
//Save Data
_logger.LogInformation("Save Comepleted");
await _next(context);
}
catch (Exception ex)
{
_logger.LogError($"Something went wrong: {ex.Message}");
}
}
Please note above , I am creating Logger object using var _logger = _loggerFactory.CreateLogger() method.
Logging Configuration for LoggerFactory
LoggerFactory shall use the default configuration as specified in appsetting.json or appsetting.[ENV].json.
This default configuration will be loaded by .NET Core’s default host builder i.e CreateDefaultBuilder loads these default settings and makes it available for logger factory and other providers etc.
Example:
{
"Logging": {
"LogLevel": {
"Default": "Information",
}
},
"AllowedHosts": "*",
"ConnectionStrings": {
"EmployeeDB": "Server=localhost\\SQL;Database=master;Trusted_Connection=True;"
}
}
Above produces below logging,

If you use Log Level as ‘Warning’ then LoggFactory will honor those settings. LoggerFactory settings will be managed by CreateDefaultBuilder by default unless you specify a different setting explicitly.
FYI CreateDefaultBuilder in ASP.NET Core is defined as below in Program.cs
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
Additionally, you get out of the box using Middleware Item templates available in Visual Studio-Right Click on Project-Add New Item and search with Middleware in item list as shown below,
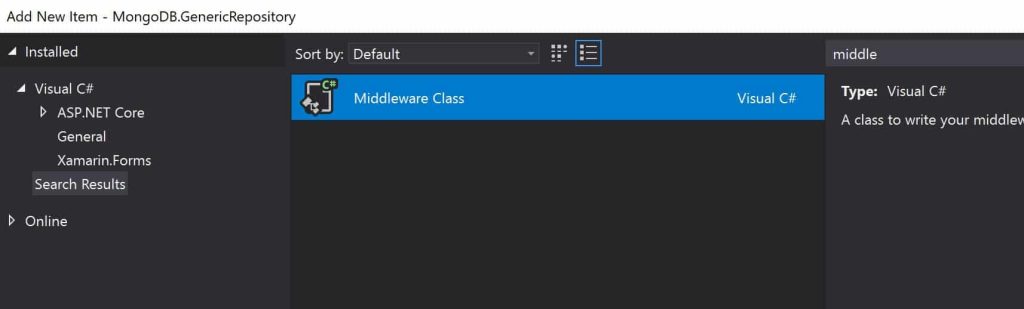
Middleware extension method
Use below extension method presents the middleware through IApplicationBuilder.
public static class GlobalCustomMiddleware
{
public static void UseGlobalCustomMiddleware(this IApplicationBuilder app)
{
app.UseMiddleware<CustomMiddleware>();
}
}
Finally, use the below code to enable middleware using UseGloablCustomMiddleware() method in the API pipeline,
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseGlobalCustomMiddleware();
app.UseHttpsRedirection();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
References:
That’s All! Happy Coding!
Do you see any improvement to the above code? Do you have any suggestions or thoughts? Please sound off your comments below.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
This post helped me out a lot!
Thanks Andre. Glad it helped you !