Making a PowerShell POST request with body – Guidelines
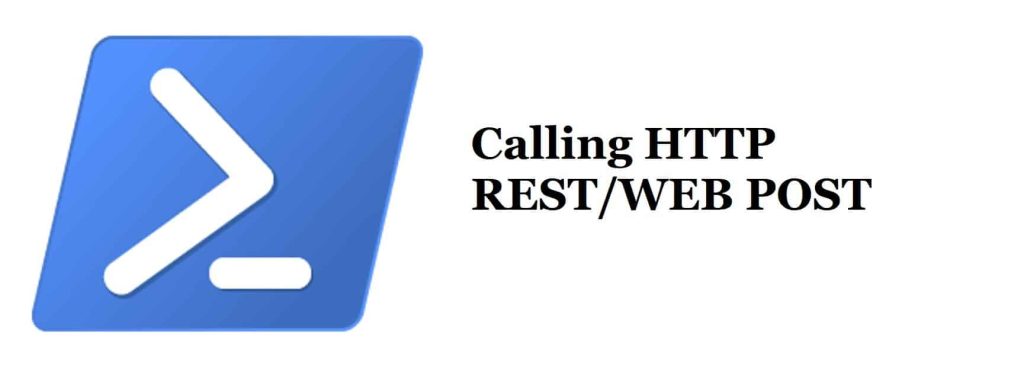
Today in this article, we shall learn how to Make PowerShell POST request with body parameters using easy-to-understand examples.
Please note that we can use Invoke-WebRequest or Invoke-RestMethod to achieve the same.
In PowerShell, you can use the Invoke-RestMethod
or Invoke-WebRequest cmdlet to send a POST request with a simple object or array as the request body.
One can easily use these cmdlets to create powerful PowerShell-based API or PowerShell Web Services calls. This CMDLet helps you write PowerShell REST Client-side code.
We shall cover the below examples,
PowerShell POST request with body
Below is the expected body parameter in the form of JSON,
{
"siteUrl": "https://www.thecodebuzz.com",
"email": "infoATthecodebuzz.com"
}
We shall use Invoke-RestMethod to use the above request body and send it in the POST body parameter,
Below is an equivalent body parameter example in PowerShell,
$body = @{
"siteUrl" ="https://www.thecodebuzz.com"
"email" = "infoATthecodebuzz.com"
}
Below is the POST method example using Invoke-RestMethod,
Invoke-RestMethod -Method 'Post' -Uri $url -Body ($body|ConvertTo-Json) -ContentType "application/json"
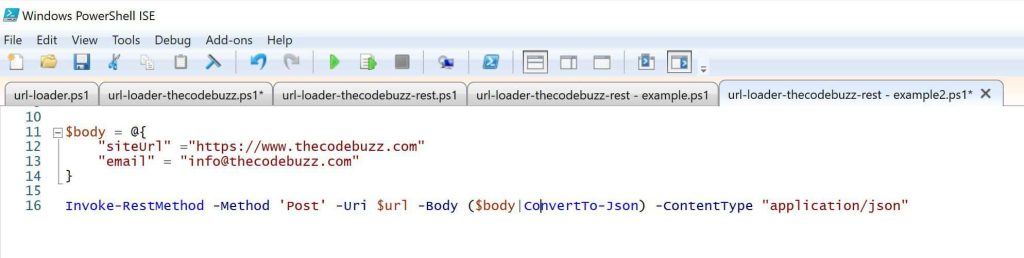
Below is the POST method example using Invoke-WebRequest,
Invoke-WebRequest -Method 'Post' -Uri $url -Body ($body|ConvertTo-Json) -ContentType "application/json"
PowerShell POST request with the body with Headers
Powershell requests with headers can be defined as below,
$headers = @{
'Content-Type'='application/json'
'apikey'='7bd43dc4216e15971'
}
Below is the Powershell POST method example with Headers,
Invoke-RestMethod -Method 'Post' -Uri $url -Body ($body|ConvertTo-Json) -Headers $headers -ContentType "application/json"
Send Array in POST Request- Using Simple Array in request Body using Powershell
One can use Array in request Body using Powershell. We can easily send Array Data in REST API.
When sending an array in the request body, you need to convert it to JSON format.
Here we need to use $(..) to represent an array.
Example 1
I have a sample JSON with an array example as below,
{
"siteUrl": "https://www.thecodebuzz.com",
"email" : "infoATthecodebuzz.com",
"itemList": [
"Item1",
"Item2",
"Item3"],
"details":[
{
name:"test",
id: "213124"
}]
}
The PowerShell request body for Array looks as below,
$body = @{
'siteUrl' = "https://www.thecodebuzz.com"
'urlList'= @(
"Item1",
"Item2",
"Item3")
}
Here is the request format,
Invoke-RestMethod -Method 'Post' -Uri $url -Body ($body|ConvertTo-Json) -Headers $headers -ContentType "application/json"
Below is the POST method example using Invoke-WebRequest,
Invoke-WebRequest -Method 'Post' -Uri $url -Body ($body|ConvertTo-Json) -Headers $headers -ContentType "application/json"
Example 2
# Sample array of strings $array = @("A", "B", "C") # Convert the array to JSON format $jsonBody = $array | ConvertTo-Json # API endpoint URL $apiUrl = "https://www.thecodebuzz.com/api/" # Send the POST request with the array as the request body $response = Invoke-RestMethod -Uri $apiUrl -Method Post -Body $jsonBody -ContentType "application/json" # Display the response $response
Send Array in POST Request
Using Array (key-Value pairs) in request Body using Powershell
$body = @{
‘siteUrl’ = “https://www.thecodebuzz.com”
‘urlList’= @(
{“Item1″=”value1”},
{“Item2″=”value2”},
{“Item3″=”value3”})
}
Here is the POST example request format,
Invoke-RestMethod -Method 'Post' -Uri $url -Body ($body|ConvertTo-Json) -Headers $headers -ContentType "application/json"
Differences between Invoke-RestMethod
Vs Invoke-WebRequest
Invoke-RestMethod
Invoke-RestMethod
efficiently deal with XML and JSON results.
It doesn’t support straight HTML(except XML-compliant HTML) while Invoke-WebRequest
efficiently deal with straight Web/HTML results.
For additional differences and guidelines, please visit this article.
For more details on handling array or JSON please refer to the below article,
HTTP/WebRequest using JSON body with array or list
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
{
“indices”: [“identities”],
“query”: {
“query”: “created:[now-24h TO now]”
}
}
if I need to pass like this data
something similar to this should work
$body = @{
‘indices’= @(“identities”)
‘query’ = @{‘query’ = “`”$nativeIdentity`””}
}
$body = $body | convertto-json
cool…how about if the body is a ZIP file ?
tks
Hi grrlgd, thanks for your query! it should works just fine , if you send streams if bytes as string or custom type for a zip content. Hope it helps.