PowerShell Script- Using JSON Array or List
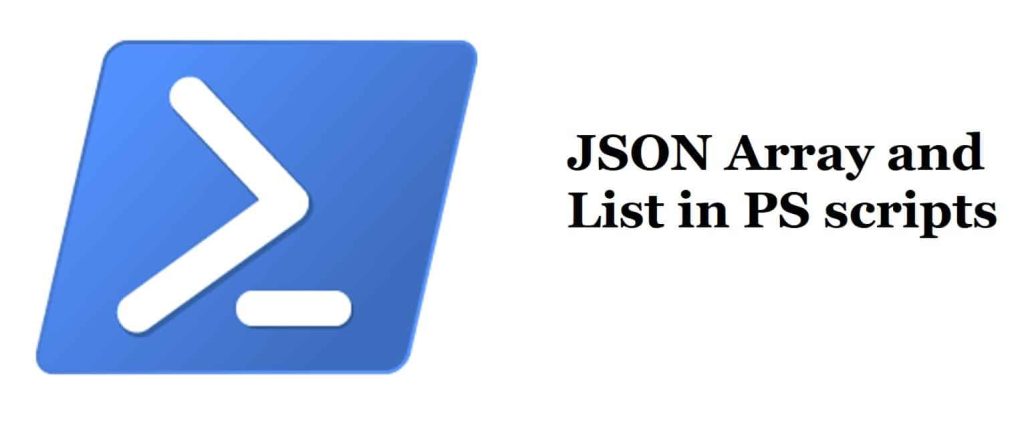
Today in this article, we shall see how to generate or how to use Use JSON array fields in a PowerShell script.
This conversion is often needed when you are trying to perform GET or POST requests with body parameters to understand examples.
Let’s use the below sample JSON which we want to represent/Generate in the PowerShell script.
Sample JSON Input
{
"siteUrl": "https://www.thecodebuzz.com",
"email": "[email protected]",
"itemList": [
"Item1",
"Item2",
"Item3"
],
"details": [
{
"name": "test",
"id": "213124"
},
{
"name": "test2",
"id": "453534"
}
]
}
As shown above, we are dealing with two types of array i.e itemList and details.
We can very much create a Powershell script equivalent of the above JSON sample as below,
As we can see regular Array can be represented as,
'urlList'= @(
"item1",
"item2",
"item3",
"item4"
)
For complex array is represented(representing object or complex type) as below,
'details'=@(
@{
"name"= "test"
"id"= "213124"
},
@{
"name"= "test2"
"id"= "64564"
}
)
ConvertFrom-Json -InputObject $json
The above commands will convert JSON to Windows PowerShell objects.

Below is the final PowerShell script,
$body = @{
'siteUrl' ="https://www.thecodebuzz.com"
'urlList'= @(
"item1",
"item2",
"item3",
"item4"
)
'details'=@(
@{
"name"= "test"
"id"= "213124"
},
@{
"name"= "test2"
"id"= "64564"
}
)
}
ConvertTo-Json -InputObject $body
The above utility method i.e ConvertTo-Json will produce the JSON string that we started with above confirming our representation in the form of ps script is correct.
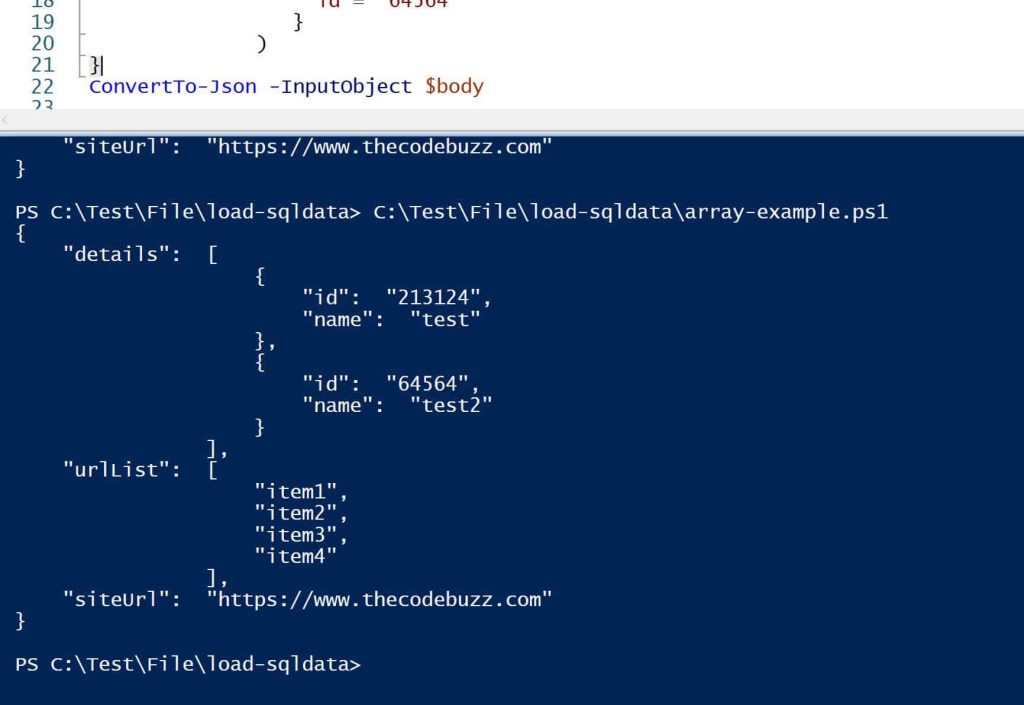
Send Array in POST Request
PowerShell JSON Array examples as below.
Example 1
# Sample array of strings $array = @("A", "B", "C") # Convert the array to JSON format $jsonBody = $array | ConvertTo-Json # API endpoint URL $apiUrl = "https://www.thecodebuzz.com/api/" # Send the POST request with the array as the request body $response = Invoke-RestMethod -Uri $apiUrl -Method Post -Body $jsonBody -ContentType "application/json" # Display the response $response
Example 2
$body = @{
'siteUrl' = "https://www.thecodebuzz.com"
'urlList'= @(
"Item1",
"Item2",
"Item3")
}
Here is the request format,
Invoke-RestMethod -Method 'Post' -Uri $url -Body ($body|ConvertTo-Json) -Headers $headers -ContentType "application/json"
References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.