MongoDB – Convert DateTime to C# DateTime
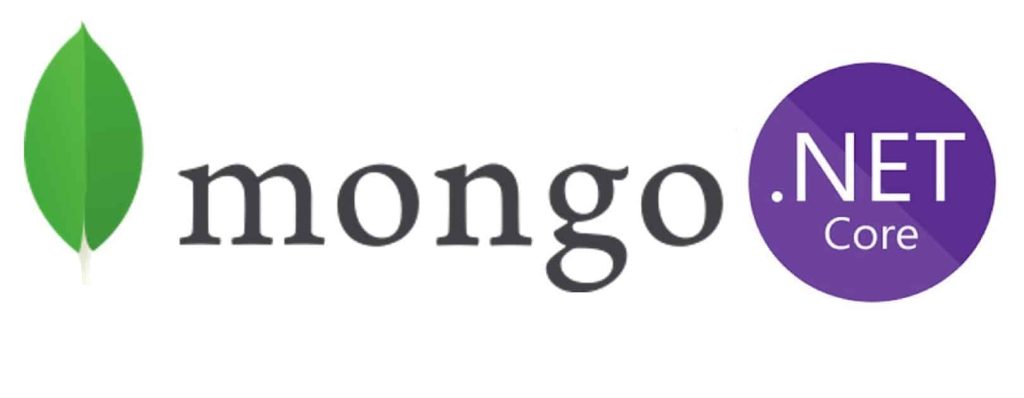
Today in this article will see how to Convert MongoDB DateTime to C# DateTime object using C# MongoDB driver in .NET or .NET Core based application.
Getting started
Please create any .NET/.NET Core application.
Please add the MongoDB driver NuGet package using the Nuget Package manager.
PM> Install-Package MongoDB.Driver -Version 2.9.3
MongoDB bascailly supports ISO DateTime format. If you are writing the code in C# then you need not have to format a lot as it support the format with basic changes in the code.
Here below is actual sample schema or document where i get the Date in the format
yyyy-MM-dd'T'HH:mm:ss.fff'Z
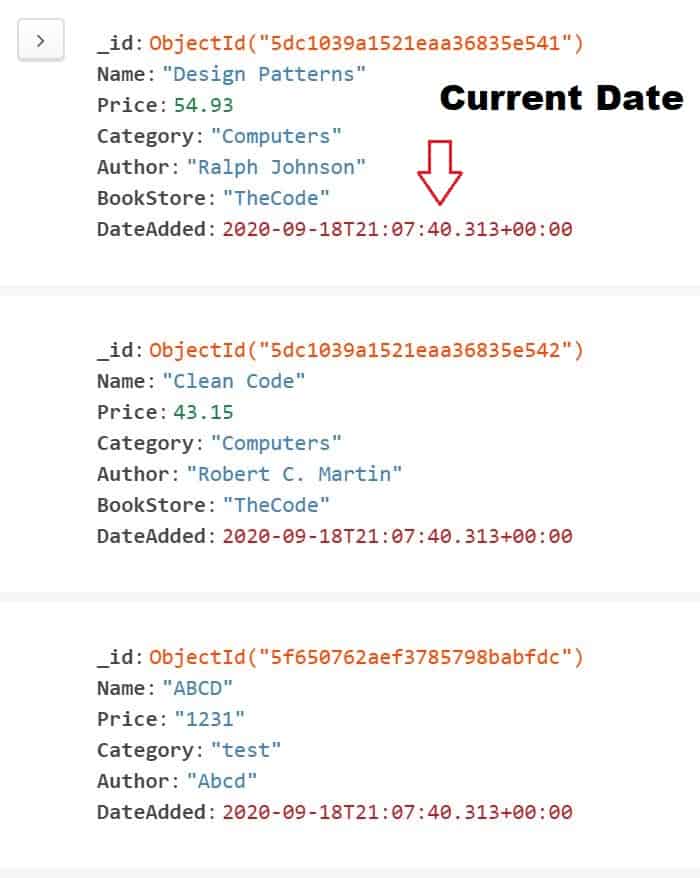
Let’s see how to convert the above date format to consume in C# code in the format of a DateTime object.
Solution 1

The above code uses BsonDocument as a generic type. I am using GetElement to get the required data.
Above code easily format to DateTime object. Once you have the DateTime object you can verify convert the format to any format of your choice.
Solution 2
Please use Convert.DateTime method to convert date time value easily into C# DateTime.

Solution 3
Similarly, if you have a raw date from another sources simply pass it to Convert.ToDate() method for the required conversion.
string dateInput = "2020-11-12T22:18:18.515Z";
DateTime dateAdded = Convert.ToDateTime(dateInput);
If you have any custom type defined then the property with DateTime property can be mapped directly without having to do any conversion.
Below logic will print all supported format. This is just for your references purpose only.
foreach (var customString in DateTimeFormatInfo.CurrentInfo.GetAllDateTimePatterns('d'))
{
Console.WriteLine(" {0}", customString);
}
Formats example :
- M/d/yyyy
- M/d/yy
- MM/dd/yy
- MM/dd/yyyy
- yy/MM/dd
- yyyy-MM-dd
- dd-MMM-yy
References:
That’s all! Happy coding!
Does this help you fix your issue?
Do you have any better solutions or suggestions? Please sound off your comments below.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.