Using MongoDB in .NET Core Console Application

In this article, we will see how to get started using MongoDB in C# Console Application
We shall be using the MongoDB database as the NoSQL database instance. We shall see and leverage the usage of Mongo DBContext object using Dependency injection.
Today in this article, we will cover below aspects,
We shall create a MongoDB context object which will resemble EF Core generated DBContext scaffolding (Entity Framework is an ORM framework for relational DB)
Here we shall create a Context class which will help us to create abstraction around Mongo objects.
Getting Started
Create a .NET Core Console application
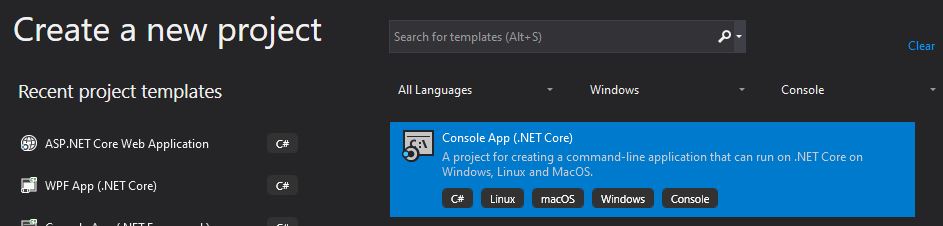
Please add the MongoDB driver NuGet package using the Nuget Package manager or PMC command,
PM> Install-Package MongoDB.Driver -Version 2.10.3
OR

MongoDB Configuration
Below is the MongoDB connection configuration defined in appsettings.json.
Please add a new appsettings.json file to your console application and define the MongoDB connection string and Database name as below,
{ "MongoSettings": { "ConnectionString": "mongodb://connection string", "DatabaseName": "Book" } }
Setting up generic generic for Mongo DBContext object
Let’s create a generic HosBuilder and register the dependencies that need to be injected which is explained in detail below.
MongoDB Context in DI Container
We will set generic HostBuilder for the non-host Console application.
Please install the NuGet package from Nuget Package manager or PMC,
PM> Install-Package Microsoft.Extensions.Hosting -Version 3.1.2
Below is the Main() method implementation .
This gives you an early idea of the Interfaces and Classes we shall be creating at the end of this article.

Define MongoDBContext Interface
We shall name Mongo DBContext Interfaces as ‘IMongoBookDBContext‘,
public interface IMongoBookDBContext
{
IMongoCollection<Book> GetCollection<Book>(string name);
}
Create Mongo DBContext class
MongoDB DBContext class MongoBookDBContext will be defined as below,
public class MongoBookDBContext : IMongoBookDBContext
{
private IMongoDatabase _db { get; set; }
private IMongoClient _mongoClient { get; set; }
public IClientSessionHandle Session { get; set; }
public MongoBookDBContext(IOptions<Mongosettings> configuration)
{
_mongoClient = new MongoClient(configuration.Value.Connection);
_db = _mongoClient.GetDatabase(configuration.Value.DatabaseName);
}
public IMongoCollection<T> GetCollection<T>(string name)
{
if(string.IsNullOrEmpty(name))
{
return null;
}
return _db.GetCollection<T>(name);
}
}
So DBContext class will help to establish an actual connection, providing the database collection as required.
Model Entity as Book
Based on the schema this is how the model class has been defined as below,
public class Book { [BsonId] [BsonRepresentation(BsonType.ObjectId)] public string Id { get; set; } public string Name { get; set; } public decimal Price { get; set; } public string Category { get; set; } public string Author { get; set; } }
Define MyApplication class
So finally we are all set with Models and types required for us to connect and perform our CRUD operation.

Performing CRUD operations
We are using the FindSync method which is an extension method.
Below I am using ObjectId as a unique identifier to read the details about the Book.
public async Task GetBookDetails()
{
var objectId = new ObjectId("5dc1039a1521eaa36835e541");
FilterDefinition <Book> filter = Builders<Book>.Filter.Eq("_id", objectId);
var result = await _dbCollection.FindAsync(filter).Result.FirstOrDefaultAsync();
Console.WriteLine(result);
}
Let’s execute the Read operation API and verify the results,

That’s all this was very much basics on connecting the MongoDB database using MongoDB Driver C# code.
You can very much extend the above application for other CRUD operations like Create, Update, or Delete.
Do you need further improvement to this codebase ??
Please use the Repository pattern around MongoDB as discussed in the below implementation,
Simple MongoDB usage without DI,
Summary
In this post, we learned how to use MongoDB in a .NET Core Console application. We learned to create a Context definition for the existing No-SQL database and then leveraged the IoC container registering the DBContext objects, and other services, and performing CRUD operations.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.