Http GET, POST, PUT and DELETE example using HttpClientFactory
In today’s post, we will see the HttpClientFactory HTTP GET POST examples. We shall create a basic or named HttpClient instance.
In this instance, we shall use HTTP GET, POST PUT, or DELETE methods.
Today in this article, we will cover below aspects,
In our previous article, we already also looked at approaches to create HTTPClient requests in ASP.NET Core API.
Let’s look at a few examples to invoke HTTP GET and POST methods using HttpClientfactory.
As discussed in the previous article I have already setup the named HttpClientclientFactory to create the required HttpClient instance as below,
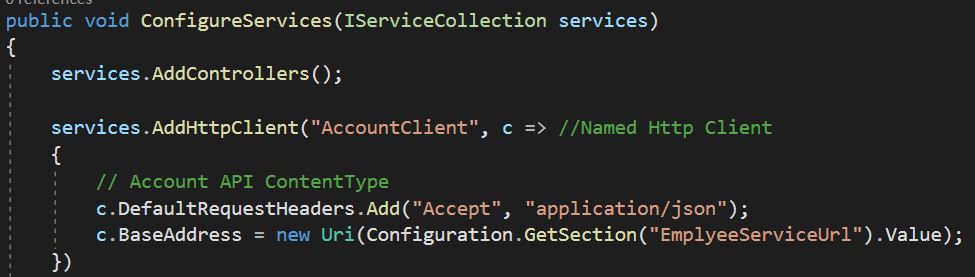
Calling the GET method using HttpClientFactory
Below is a sample GET method that shows creating a named instance of HttpClient using HttpClientFactory and invoking the GET method asynchronously.
//GET: api/Accounts/PersonalAccounts/1234
[HttpGet]
[Route("PersonalAccounts")]
public async Task<IActionResult> GetAccountDetails(int employeeId)
{
var client = _clientFactory.CreateClient("AccountClient");
var response = await client.GetAsync($"api/accounts/PersonalAccounts/{employeeId}");
if (response.IsSuccessStatusCode)
{
return Ok(response.Content.ReadAsStreamAsync().Result);
}
else
{
return StatusCode(500, "Something Went Wrong! Error Occured");
}
}
In the above example, we are using the IHttpClientFactory instance to create a client. Using generated client we invoke the GET call for the given Uri and return the result.
Calling the POST method using HttpClientFactory
Below is a sample POST method example. Here I am creating a named instance of HttpClient using HttpClientFactory and invoking the POST method asynchronously.
Note: Below samples are shown using direct usage of post-call in Controller. Ideally please structure your code considering the separation of code.

Ideally, the POST method should also return the location of newly created resources as per REST guidelines.
Calling the PUT method using HttpClientFactory
Below is a sample PUT method using HttpClientFactory and invoking the Put method asynchronously.
//PUT: api/Accounts/1234
[HttpPut]
public async Task<IActionResult> Put(int employeeId, Customer customer)
{
CusotmerDetails employeeDetails = null;
var client = _clientFactory.CreateClient("AccountClient");
HttpContent httpContent = new StringContent(JsonConvert.SerializeObject(customer)
, Encoding.UTF8, "application/json");
HttpResponseMessage response = await client.PutAsync($"api/accounts/{employeeId}", httpContent);
if (!response.IsSuccessStatusCode)
{
return StatusCode(500, "Something Went Wrong! Error Occured");
}
employeeDetails = JsonConvert.DeserializeObject<CusotmerDetails>(response.Content.ReadAsStringAsync().Result);
return Ok(employeeDetails);
}
Calling the DELETE method using HttpClientFactory
Below is a sample example for DELETE using HttpClientFactory. Below we are using HttpClientFactory and invoking the method asynchronously.
//DELETE: api/Accounts/1234
[HttpDelete]
public async Task<IActionResult> Delete(int employeeId)
{
CusotmerDetails employeeDetails = null;
var client = _clientFactory.CreateClient("AccountClient");
HttpResponseMessage response = await client.DeleteAsync($"api/accounts/{employeeId}");
if (!response.IsSuccessStatusCode)
{
return StatusCode(500, "Something Went Wrong! Error Occured");
}
employeeDetails = JsonConvert.DeserializeObject<CusotmerDetails>(response.Content.ReadAsStringAsync().Result);
return Ok(employeeDetails);
}
References:
That’s all! Happy Coding!
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.