NCache Distributed Cache in C#.NET with Examples
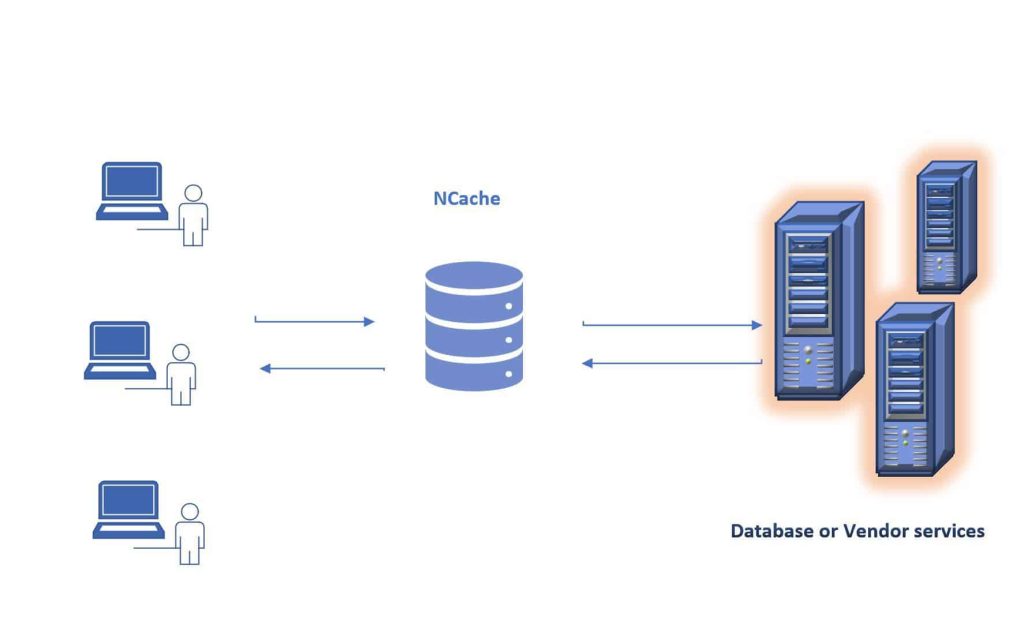
Today in this article, we will see how to use distributed Cache Using NCache in C#.NET with examples. We will also see how to leverage the IDistributedCache interface for performing cache operations using NCache.
Today in this article, we will cover below aspects,
NCache is a distributed in-memory database.
NCache is one of the Open Source in-memory, distributed caches for .Net Core which is extremely scalable, and caches application data quickly.
In our previous article, we looked at how to leverage the ICache interface which is provided by NCache. For more details please visit the below article,
Using IDistributedCache for NCache
We can leverage a Microsoft-provided IDistributableCache interface to leverage the Cache capability in the applications.
This interface comes as caching abstraction extension which can be used and plugged into any cache of your choice like Redis or NCache solutions etc.
This Interface exposes the below methods for performing get or update or deletes operations on the cache,
- Get and GetAsync
- Set and SetAsync
- Refresh and RefreshAsync
- Remove and RemoveAsync
In today’s article, however, we will cover, how to use the ICache interface which is very rich and provides a lot of capabilities compare to the IDistributableCache interface.
Let’s brief about on the few benefits of NCache, which we learned in the previous article,
Benefits of NCache
- Using NCache lets you access the data more quickly to lower the response time from an external system.
- We can utilize NCache to scale Applications by removing performance constraints associated with data storage and databases.
- NCache is an efficient way of getting the data, especially frequently needed information and their access.
- NCache can be used HttpBasedCaching, In-Memory Caching, or Distributed Caching.
Getting started
Before we get started, please make sure you have the NCache cache server setup configured. For this demonstration, I am using local demoCache as a Cache.
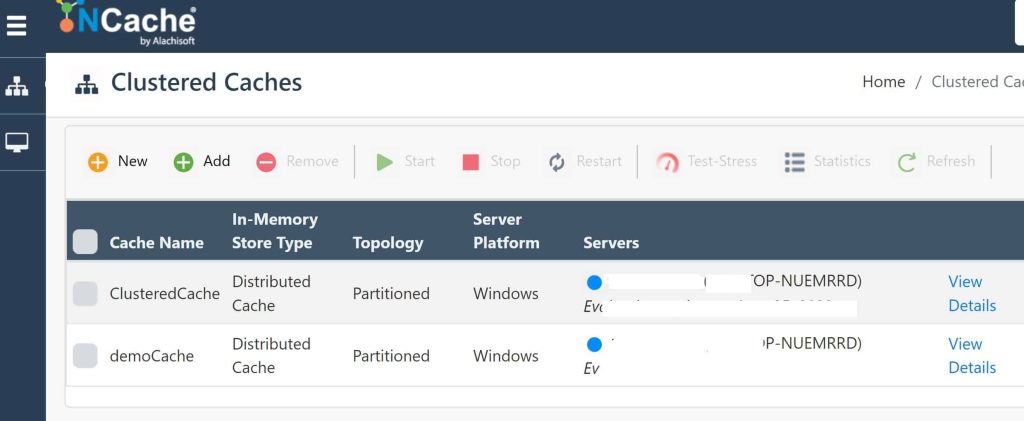
Prerequisites:
- Please make sure NCache is installed and configured correctly on the server.
- Please make sure to Configure the server/cluster in client.ncconf.
When you install the NCache Nuget package, it adds the Client.ncconf and Config.ncconf files to you local application.
–Client.ncconf contains the information about the cache servers.–Config.ncconf that is copied locally is used for local inproc cache and inproc client caches.Also config.ncconf must also contain the name for the clustered cache which this client cache is a part of the applicaiton
Create ASP.NET Core API/ MVC Application
Create .NET 3.1 or 5 API or ASP.NET Core MVC applications.
Set Up Environment for AspNetCore.Session.NCache
Please install the NCache NuGet package version as required,
For OpenSource:
Install-Package NCache.Microsoft.Extensions.Caching.OpenSource
For the Enterprise/Professional version, please install the below version as required,
Install-Package NCache.Microsoft.Extensions.Caching
OR
Install-Package NCache.Microsoft.Extensions.Caching.Professional
Using AddNCacheDistributedCache in the API pipeline
Update the ConfigureServices methods with the below-highlighted code,
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddScoped<IEmployeeRepository, EmployeeRepository>();
services.AddScoped<INCacheCacheService, NCacheCacheService>();
services.AddDbContext<EmployeeContext>(options =>
{
options.UseSqlServer(Configuration.GetConnectionString("EmployeeDB"),
sqlServerOptionsAction: sqlOptions =>
{
sqlOptions.EnableRetryOnFailure();
});
});
//Lets you Use NCache as Distributed cache in the applicaiton
services.AddNCacheDistributedCache(configuration =>
{
configuration.CacheName = Configuration["NCacheConfig:CacheName"];
});
}
We will see implementation details for INCacheCacheService and IEmployeeRepository below.
INCacheCacheService is defined as below,
public interface INCacheCacheService
{
T Get<T>(string key);
T Set<T>(string key, T value);
}
IDistributedCache using Constructor injection
Below we are injecting the IDistributedCache interface through constructor injection.
Here is a sample implementation INCacheCacheService interface using IDistributedCache.
public class NCacheCacheService : INCacheCacheService
{
private readonly IDistributedCache _cache;
public NCacheCacheService(IDistributedCache cache)
{
_cache = cache;
}
public T Get<T>(string key)
{
var value = _cache.GetString(key);
if (value != null)
{
return JsonSerializer.Deserialize<T>(value);
}
return default;
}
public T Set<T>(string key, T value)
{
_cache.SetString(key, JsonSerializer.Serialize(value));
return value;
}
..
}
The following sample code snippet is getting the Employee detail based on the cache key, where
- If an item exists in the cache, the item will be returned straight from the cache, avoiding the heavy call to the database.
- If the item doesn’t exist in the cache, the item will be fetched from the actual database the very first time.
- Also, at the same time cache is updated with that fetched item.
- For any subsequent calls on the same key/item, data will be provided from NCache.
Below is a sample implementation for the Repository class.
public class EmployeeRepository : IEmployeeRepository
{
private readonly EmployeeContext _context;
private readonly INCacheCacheService _nCache;
public EmployeeRepository(EmployeeContext context, INCacheCacheService nCache)
{
_context = context;
_nCache = nCache;
}
public Employee GetEmployee(int? employeeID)
{
var cachedId = _nCache.Get<Employee>(employeeID.ToString());
if (cachedId != null) return cachedId;//return cache saved ids
else
{
//If Cache doesnt exist then get the data from actual source like database
//service or external vendor sevrvices etc.
Employee emp = _context.Employee.Find(employeeID.ToString());
//Lets now cache this object
if(emp!=null)
_nCache.Set<Employee>(employeeID.ToString(), emp);
//return the same object if accessed first time within timeout period
return emp;
}
}
..
..
}
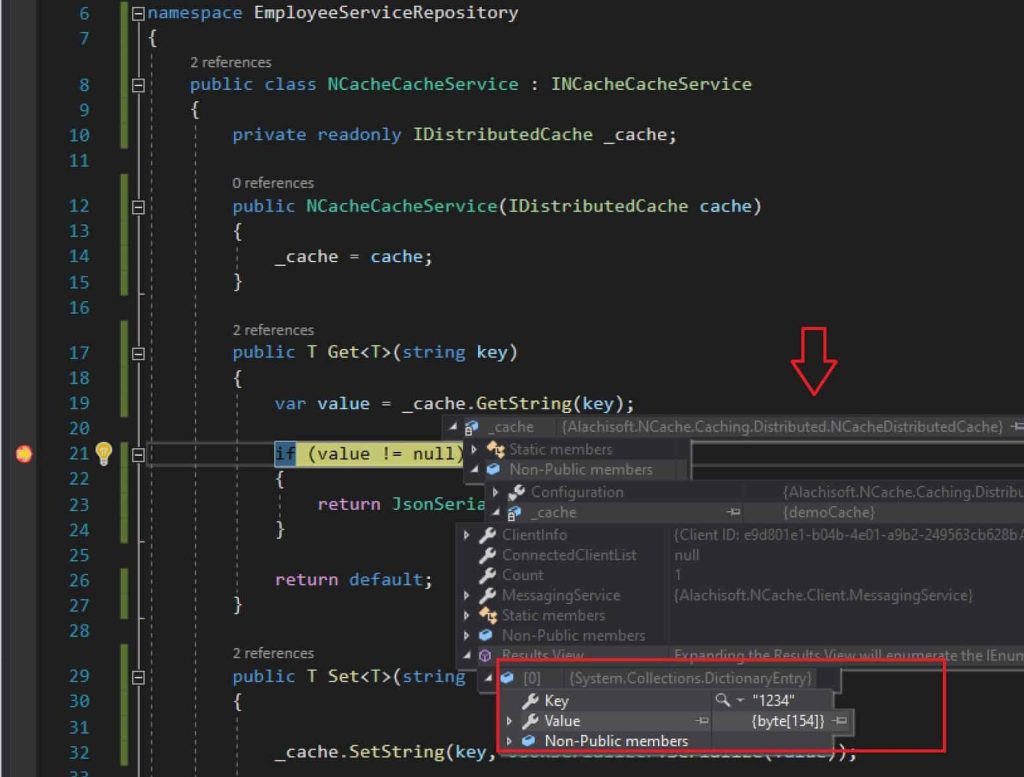
If you are interested to leverage the ICache interface from NCache using dependency injection, then please visit this article for more details.
Useful References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.