Getting started with NCache In .NET Core – Guidelines
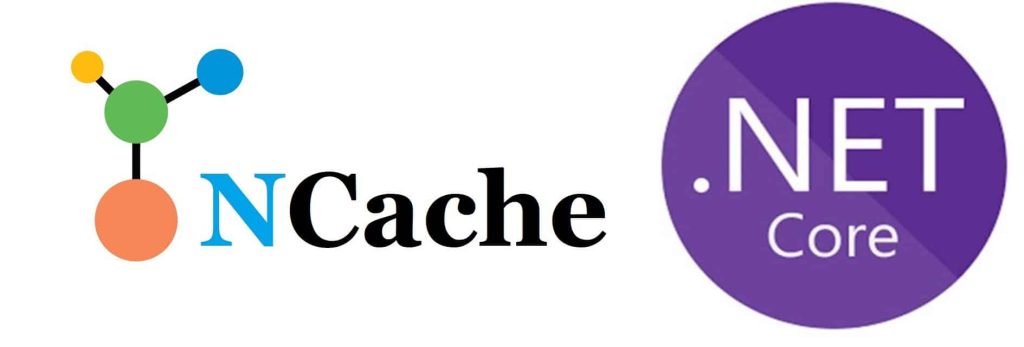
Today in this article, we will see how to get started with NCache In ASP.NET Core with examples.
Application and performance are critical, particularly the end-user experience, which may be achieved by focusing on application usage and its resource delivery approaches.
One such strategy is to implement and optimize caching in the application stack.
This end-user experience is not limited to UI. Every layer of application has equal requirements for better response time.
Today in this article, we will cover below aspects,
NCache is one of the Open Source in-memory, distributed caches for .Net Core. It’s extremely scalable, and it caches application data quickly.
It can be effectively utilized to save money on database trips.
ICache Vs IDistributable Cache
We can leverage any of the interfaces like ICache( NCache) or Microsoft-provided IDistributableCache to leverage the Cache details.
IDistributableCache provides very basic functionalities like the ability to get, update, or delete the cache.
ICache interface is a rich interface that provides below a few additional features along with basic (get, update, or delete) operation supports,
- Supports bulk Get operation and supports retrieving the objects from the cache for the given keys as key-value pairs.
- Support bulk Add operation and support Adding a dictionary of cache keys.
- Bulk delete support to remove the specified list of keys from the cache.
- Provide Lock and unlock support for an item in the cache.
Advanced NCache Features
Please see below NCache’s advanced caching features as stated below but not limited to,
- Pub/Sub Messaging
- Sync Cache with Database
- Search Cache with SQL Queries
- Data Relationships in the Cache
For more details, please visit: NCache scalability in .NET
In today’s article, however, we will cover, how to use the ICache interface which is very rich and provides a lot of capabilities compared to the IDistributableCache interface.
Benefits of NCache
- Using NCache lets you access the data more quickly to lower the response time from an external system by a service provider.
- We can utilize NCache to scale Applications by removing performance constraints associated with data storage and databases.
- NCache is an efficient way of getting the data, especially in the case of frequently needed information access.
- NCache can be used in HttpBasedCaching, In-Memory Caching, or Distributed Caching.
Getting started
Before we get started, please make sure you have the NCache cache server setup configured.
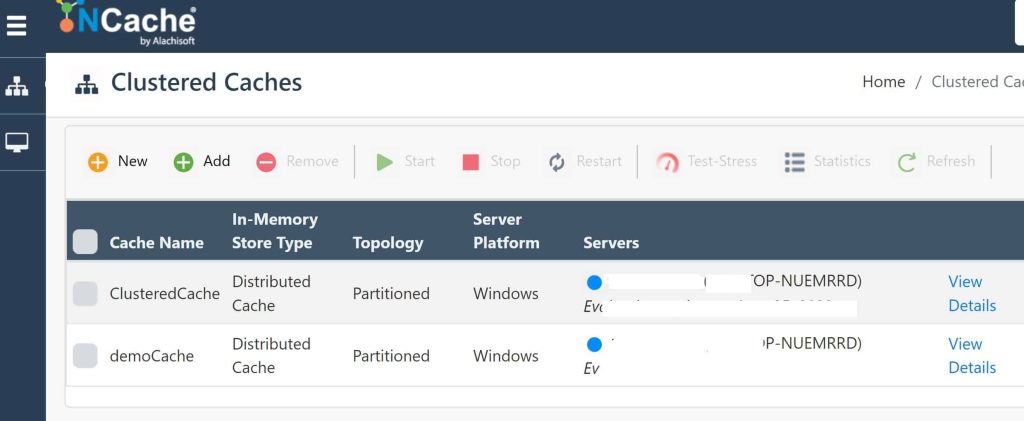
Prerequisites:
- Please make sure you have NCache is installed and configured correctly on the server.
- Please make sure to Configure the cache cluster in client.ncconf.
When you install the NCache Nuget package, it adds the Client.ncconf and Config.ncconf files to you local application.
–Client.ncconf contains the information about the cache servers.–Config.ncconf that is copied locally is used for local inproc cache and inproc client caches.Also config.ncconf must also contain the name for the clustered cache which this client cache is a part of the applicaiton
Create ASP.NET Core API/ MVC Application
- Create .NET 3.1 or 6 API or ASP.NET Core MVC applications.
- Set Up Environment for AspNetCore.Session.NCache
- Please install the NCache NuGet package version as required,
For OpenSource:
Install-Package NCache.Microsoft.Extensions.Caching.OpenSource
For the Enterprise/Professional version, please install the below version as required,
Install-Package NCache.Microsoft.Extensions.Caching
OR
Install-Package NCache.Microsoft.Extensions.Caching.Professional
Using NCache Caching configuration
For ASP.Net Applications, NCache provides a custom function for configuring a Distributed cache. We can add the Cache configuration in the app settings of that application which tells about the cache name.
Here’s a sample configuration
"NCacheConfig": {
"CacheName": "demoCache", //Replace "demoCache" with the name of your cache
"EnableLogs": false,
"EnableDetailLogs": false,
"ExceptionsEnabled": false,
"OperationRetry": 0,
"operationRetryInterval": 0
}
Configurations like EnableLogs, EnableDetailLogs, and ExceptionsEnabled are optional. Use them only if they are needed.
Also, an option like OperationRetry and OperationRetryInterval uses the default value is 0 if not specified.
Once the cache configuration is mentioned in Startup.cs, use the CacheManager.GetCache () method to set NCache as the default cache for storing objects.
services.AddScoped<ICache>(p => { ICache cache = CacheManager.GetCache("democache"); return cache; });
NCache is now the underlying cache for the ICache interface.
The following code snippet is used to get the Employee detail based on the cache key, where if there is no item existing in the cache, the item is fetched from the actual database.
Below is the code,
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddScoped<IEmployeeRepository, EmployeeRepository>();
services.AddScoped<INCacheCacheService, NCacheCacheService>();
services.AddDbContext<EmployeeContext>(options =>
{
options.UseSqlServer(Configuration.GetConnectionString("EmployeeDB"),
sqlServerOptionsAction: sqlOptions =>
{
sqlOptions.EnableRetryOnFailure();
});
});
//Lets you inject ICache interface through Dependency injection
services.AddScoped<ICache>(p =>
{
ICache cache =
CacheManager.GetCache(Configuration["NCacheConfig:CacheName"]);
return cache;
});
//Lets you Use NCache as Distributed cache in the applicaiton
services.AddNCacheDistributedCache(configuration =>
{
configuration.CacheName = Configuration["NCacheConfig:CacheName"];
});
}
We will see implementation details for INCacheCacheService and IEmployeeRepository below.
NCache using ICache interface
Let’s use ICache as the underline interface for performing Cache operation. This interface contains the services and methods that are used to perform operations on the cache.
Below is the basic implementation of the interface method.
Above we have registered the implementation of ICache using a wrapper interface i.e. INCacheCacheService.
Below is a simple Wrapper interface definition for INCacheCacheService
INCacheCacheService is defined as below,
public interface INCacheCacheService
{
T Get<T>(string key);
T Set<T>(string key, T value);
}
Below we are injecting the ICache interface through constructor injection.
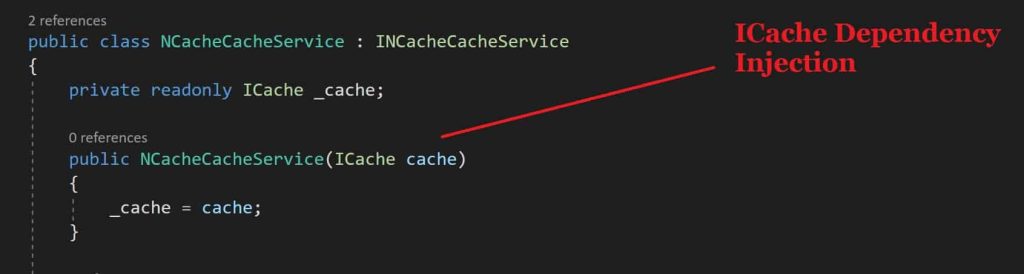
Here is a sample implementation INCacheCacheService interface using ICache.
public class NCacheCacheService : INCacheCacheService
{
private readonly ICache _cache;
public NCacheCacheService(ICache cache)
{
_cache = cache;
}
public T Get<T>(string key)
{
var value = _cache.Get<string>(key);
if (value != null)
{
return JsonSerializer.Deserialize<T>(value);
}
return default;
}
public T Set<T>(string key, T value)
{
_cache.Insert(key, JsonSerializer.Serialize(value));
return value;
}
..
..
}
Using a Repository for better abstraction
Below is a sample implementation for the Repository class,
public class EmployeeRepository : IEmployeeRepository
{
private readonly EmployeeContext _context;
private readonly INCacheCacheService _nCache;
public EmployeeRepository(EmployeeContext context, INCacheCacheService nCache)
{
_context = context;
_nCache = nCache;
}
public Employee GetEmployee(int? employeeID)
{
var cachedId = _nCache.Get<Employee>(employeeID.ToString());
if (cachedId != null) return cachedId;//return cache saved ids
else
{
//If Cache doesnt exist then get the data from actual source like database
//service or external vendor sevrvices etc.
Employee emp = _context.Employee.Find(employeeID.ToString());
//Lets now cache this object
if(emp!=null)
_nCache.Set<Employee>(employeeID.ToString(), emp);
//return the same object if accessed first time within timeout period
return emp;
}
}
..
..
}
In the above code, we get the Employee detail based on the cache key.
If the item doesn’t exist in the cache, the item will be fetched from the actual database and same time will be added to the cache.
This ensures any subsequent calls to the item are addressed through the cache.
NCache cache timeout
NCache supports time-based cache expiration. You can specify the time or interval to expire the cache data.
NCache backs Absolute and Sliding Expirations. It also supports extended flexibility to use multiple default expirations on the given dataset.
References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.