How To Send SMS In A C# .NET Core Application
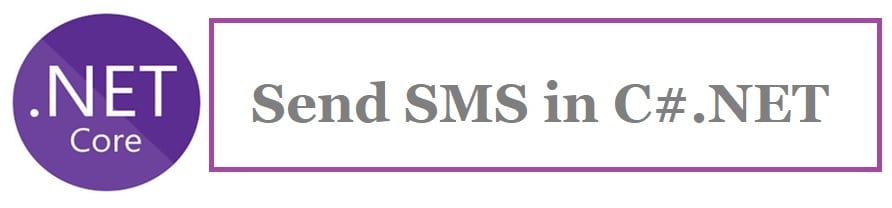
Today in this article, we will see how To Send SMS In A C# .NET Core Application.
We will cover the below aspects in the article along with Console and API examples to achieve Send SMS functionality.
Sign up for a Twilio account:
- Go to the Twilio website (https://www.twilio.com/)
- Create an account and register.
- You’ll receive a unique Account SID and Auth Token that you’ll need to authenticate your application requests.
Install Twilio package:
In your C# project, use a package manager like NuGet to install the Twilio package.
Open the NuGet Package Manager Console and run the following command:
Install-Package Twilio
Import the required namespaces
using Twilio;
using Twilio.Rest.Api.V2010.Account;
Set up the Twilio client:
You can use secured storage to store the authToken and Account SID. Once accessed, you can pass it to the Twilio init client as shown below,
TwilioClient.Init(accountSid, authToken);
Send an SMS
var message = MessageResource.Create( body: "Hello, this is a test message!", from: new Twilio.Types.PhoneNumber("YOUR_TWILIO_PHONE_NUMBER"), to: new Twilio.Types.PhoneNumber("RECIPIENT_PHONE_NUMBER") );
Please replace “YOUR_TWILIO_PHONE_NUMBER” with the phone number provided by Twilio, and “RECIPIENT_PHONE_NUMBER” with the recipient’s phone number.
Console application example:
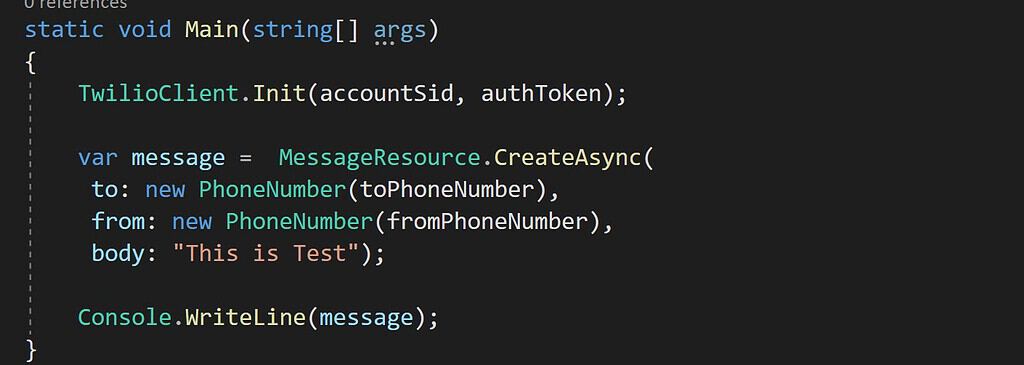
You use the configuration defined in the apsettings.json as explained in the article here Read apsettings.json Configuration without dependency injection
ASP.NET Core API example
Define the SmsSender service class as below,
public class SmsSender : ISmsSender
{
public SmsSender(IOptions<SMSConfiguration> optionsAccessor)
{
Options = optionsAccessor.Value;
}
public SMSConfiguration Options { get; } // set only via Secret Manager
public Task SendSmsAsync(string toPhonenumber, string message)
{
// Plug in your SMS service here to send a text message.
var accountSid = Options.AccountSid;
var authToken = Options.AuthToken;
TwilioClient.Init(accountSid, authToken);
return MessageResource.CreateAsync(
to: new PhoneNumber(toPhonenumber),
from: new PhoneNumber(Options.FromSourceNumber),
body: message);
}
}
ISmsSender Interface
public interface ISmsSender { public Task SendSmsAsync(string number, string message); }
Here is how we can DI ( inject) the ISmsSender service below,

Make sure you define an instance in the ConfigureServices,
public void ConfigureServices(IServiceCollection services) { services.AddControllers(); services.Configure<SMSConfiguration>(Configuration.GetSection("SMSConfiguration")); services.AddTransient<ISmsSender, SmsSender>(); }
POST request to send SMS

That’s it! Above discussed code, the snippet sends an SMS using Twilio in C# code using a Console application or other type of application.
Once you upgrade the account, you can remove the “Sent from Your Twilllio trial account” prefix from the original message.

You can customize the message body, sender’s phone number, and recipient’s phone number according to your needs. Make sure to handle any exceptions that may occur during the SMS-sending process.
If you are interested to know how to create ASP.NET API or Service application for sending SMS programmatically, Kindly see the below article for more information.
Note: Don’t forget to include the necessary error handling and ensure that you have sufficient Twilio credits or a phone number capable of sending SMS.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.