NLog – File logging in Console application .NET Core using Generic Host Builder – Part II

Today in this article, we will see how to achieve logging in Console Application using NLog in the .NET Core application.
As we know .NET Core has introduced ILogger as a generic interface for logging purposes.
This framework supported interface ILogger can be used across different types of applications like ASP.NET Core API logging, Console App logging or Windows Form app logging using built-in providers which help us logging to Console, Debug, EventSource source targets.
We also discovered that the File-based logging provider is still not available through the .NET Core framework and we need to rely on external solutions. Today we shall see how to use NLog addressing the logging requirement in the Console application.
Today in this article, we will cover below aspects,
We shall be leveraging DI( Dependency Injection) framework to DI the NLog logger object into the mini IoC Container in a .NET Core based COnsole application.
Microsoft recommends using a third-party logger framework like a Serlilog or Log4Net or NLog for high-end logging requirements like Database or File/Rolling File logging.
Getting Started
Here I am using a .NET Core Console application template,

Configuring NLog in Console Application
NLog is available as Nuget package and can be installed from NuGet Packages Manager in a give Console application,
PM> Install-Package NLog.Web.AspNetCore -Version 4.9.1
OR You can use below Nuget package
PM> Install-Package NLog.Extensions.Logging -Version 1.6.2
I found and verified above any of Nuget pacakges works fine for .NET Core Console application
You can also install any of above Nuget package using Nuget Package Manager,
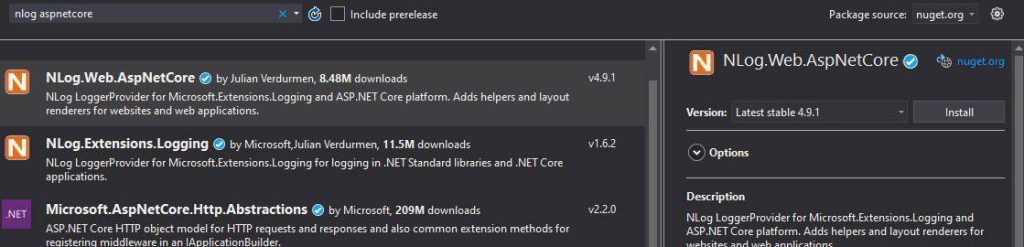
Creating Generic HostBuilder
We will be leveraging DI( Dependency Injection) framework to inject the NLog logger.
So as a first step, Let’s create generic Hostbuilder. This HostBuilder shall help us to define our DI Container too.
Please install the NuGet package from Nuget Package manager or PMC,
PM> Install-Package Microsoft.Extensions.Hosting -Version 3.1.1
The HostBuilder class is available from the following namespace,
using Microsoft.Extensions.Hosting;
Please create Generic HosBuilder and register your services and business dependencies. We shall also be registering the NLog interface.
I have created a class called ‘MyApplication‘ which can be registered as a in the IoC container.
See below implementation for HostBuilder and registration for NLog logging.
These changes can be done in the Main() method,
var builder = new HostBuilder()
.ConfigureServices((hostContext, services) =>
{
services.AddTransient<MyApplication>();
});
Register NLog logging in IoC
The next step is to register NLog in the IoC container using the ConfigureLogging method.
We can also set a minimum level of logging as defaults Example ‘Trace’ or ‘Information’ as below,
Please add below changes in the Main() method.
var builder = new HostBuilder()
.ConfigureServices((hostContext, services) =>
{
services.AddTransient<MyApplication>();
})
.ConfigureLogging(logBuilder =>
{
logBuilder.SetMinimumLevel(LogLevel.Trace);
logBuilder.AddNLog("nlog.config");
}).UseConsoleLifetime();
var host = builder.Build();
using (var serviceScope = host.Services.CreateScope())
{
var services = serviceScope.ServiceProvider;
try
{
var myService = services.GetRequiredService<MyApplication>();
myService.Run();
Console.WriteLine("Success");
}
catch (Exception ex)
{
Console.WriteLine("Error Occured");
}
}
Please add a new nLog.config file if not available using Visual Studio ->Add New Item.
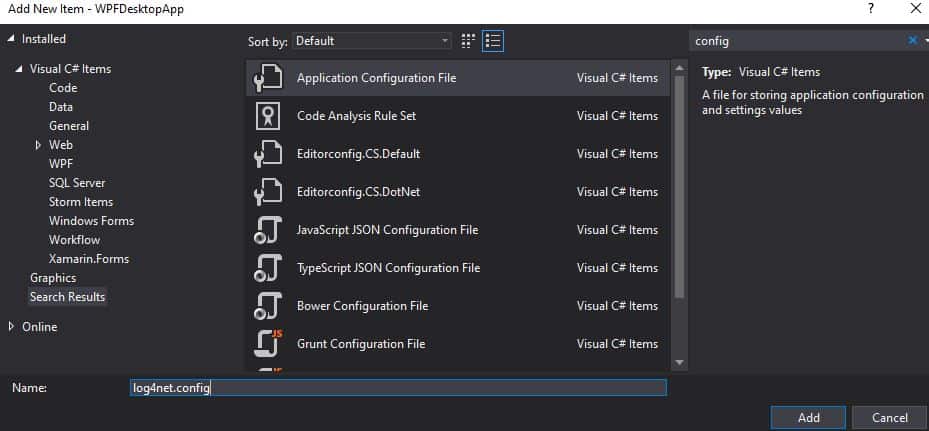
This file needs to be updated with log layout details and configuration as given below as an example.
Below is the complete implementation for IoC container implementation which you need to add to the Main() method.
All set lets now define and do dependency injection of ILogger instance within Application Class as below,

The log file shall be created in the project output directory unless specified with a custom path.
Below shows an example of file logging created by NLog,

NLog.Config File example
NLog Lets you control log level and log message structure. It also lets you control the file layout etc. if needed.
Sample NLog.config file is as below,
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<nlog xmlns="http://www.nlog-project.org/schemas/NLog.xsd"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
autoReload="true"
internalLogLevel="Info">
<!-- the targets to write to -->
<targets>
<!-- write logs to file -->
<target xsi:type="File" name="allfile" fileName="Nlog-TheCodeBuzz-${shortdate}.log"
layout="${longdate}|${event-properties:item=EventId_Id}|${uppercase:${level}}|${logger}|${message} ${exception:format=tostring}" />
</targets>
<!-- rules to map from logger name to target -->
<rules>
<!--All logs, including from Microsoft-->
<logger name="*" minlevel="Trace" writeTo="allfile" />
</rules>
</nlog>
</configuration>
References: Dependency Injection in Console App in .NET Core
Please let me know if you have any questions or suggestions. Please sound off your comments below.
Happy Coding !!
Summary
NLog helps us enabling logging in a few simple steps and addresses the high-end logging requirement .NET Core Console application and helps you logging on Console or File-based logging.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.