Python – Azure Storage Blob Download and Read

Today in this article, we will see how to perform Python – Azure Storage Blob Download or Read programmatically. We will also cover a few best practices while downloading or reading from Azure storage.
We will use the azure-storage-blob SDK package for Python.
In this comprehensive guide, we’ll explore how to read or download files from Azure Blob Storage in Python, covering the necessary steps, code examples, best practices, and potential considerations.
One can easily perform blob Storage CRUD operations like addressing the common Create, Read, Update, and Delete actions performed on blobs. However, in this article, we will cover mainly Read operations.
Overview of Azure Blob Storage
Azure Blob Storage is Microsoft’s cloud object storage solution, designed for storing and serving large amounts of unstructured data, such as documents, images, videos, and logs.
Blob Storage offers various storage tiers, access tiers, and features like versioning encryption, and lifecycle management.
Prerequisites
Before we proceed, ensure you have the following prerequisites:
- An Azure subscription: You’ll need an Azure account to access Azure Blob Storage.
- Azure Storage account: Create a storage account in the Azure portal.
- Azure Storage SDK for Python: Install the
azure-storage-blob
package using pip.
pip install azure-storage-blob
Retrieving connection string from Azure
Please follow the below steps to get the connection string from the Azure portal,
- Sign in to the Azure portal account
- Go to your storage account. Ex. vstorageaccount
- Click on the Access keys.
- Copy the Connection string value under key1
Below is how I can get the Connection string from the Azure portal,
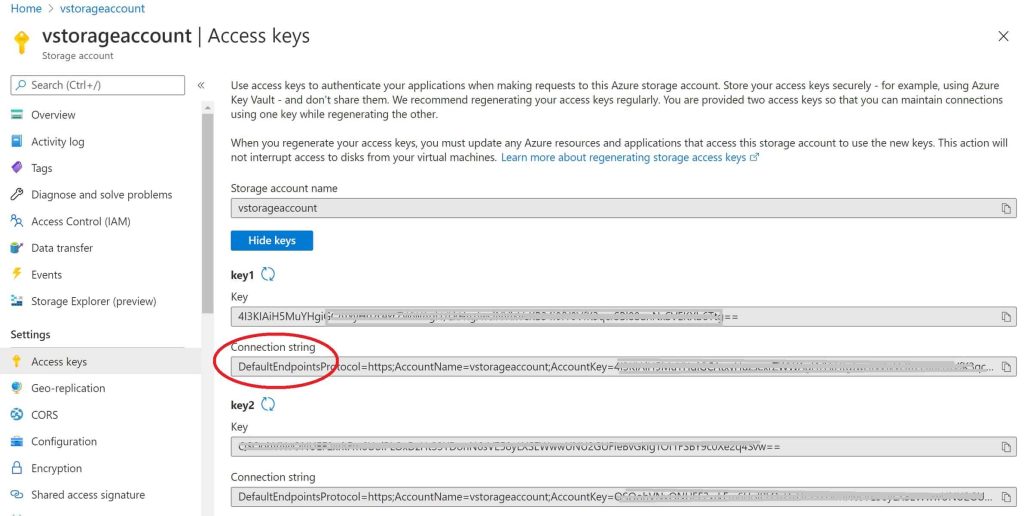
Steps to Read Files from Azure Blob Storage
To read files from Azure Blob Storage using Python, follow these steps:
Authentication: Authenticate with Azure using credentials
from azure.storage.blob import BlobServiceClient
from azure.core.exceptions import ResourceNotFoundError
# Azure Blob Storage credentials
connection_string = "connection_string"
container_name = "container_name"
blob_name = "blob_name"
# Authenticate with Azure
blob_service_client = BlobServiceClient.from_connection_string(connection_string)
....
Access Blob Container
Connect to the Blob Storage container where your files are stored.
Read data from Azure blob storage python
You can download or Read data from Azure blob storage python
Download File: Download the desired file from Blob Storage to the local filesystem.
...
# Download Blob
with open("azure_file_to_be_downloaded.txt", "wb") as download_file:
download_file.write(blob_client.download_blob().readall())
...
Read data from Azure blob storage python without download
Read data from Azure blob storage python without download can be done as below.
..
#Get Blob Client
blob_client = blob_service_client.get_blob_client(container=container_name, blob=blob_name)
# Read file contents
with open("downloaded_file.txt", "r") as file:
file_contents = file.read()
print(file_contents)
..
Sample Example
Below sample reusable function which can be used in code

The above can be used in code as below,
# Example usage
if __name__ == "__main__":
# Azure Blob Storage connection details
# Read the file content from Azure Blob Storage
file_content = read_blob(storage_connection_string, container_name, blob_name)
print("File content:", file_content)
Best Practices and Considerations
- Security: Keep your Azure credentials secure and avoid hardcoding them in your code. Consider using Azure Key Vault for storing sensitive information.
- Error Handling: Implement error handling to gracefully handle exceptions and failures during authentication, file download, and reading.
- Optimization: Optimize file downloads by using streaming Azure storage blob or asynchronous methods for large files to reduce memory consumption and improve performance.
- Monitoring: Monitor Azure Blob Storage usage, performance, and costs using Azure Monitor and Azure Storage Analytics.
- Access Control: Configure access control policies and permissions for Blob Storage containers and blobs to enforce security and compliance requirements.
Future Considerations
Looking ahead, consider these potential future enhancements and considerations:
- Integration with Azure Services: Explore integrations with other Azure services like Azure Functions, Azure Data Lake Storage, and Azure Cognitive Services for advanced analytics, processing, and insights.
- Scalability: Design your applications for scalability and performance to handle increasing data volumes and user loads.
- Automation: Automate file processing tasks using Azure Functions, Azure Logic Apps, or Azure Data Factory for seamless integration and workflow automation.
- Cost Optimization: Continuously optimize costs by leveraging Blob Storage access tiers, lifecycle management policies, and Azure Cost Management tools.
Conclusion
In this guide, we’ve learned how to read files from Azure Blob Storage using Python.
By following best practices, considering security, error handling, optimization, and future considerations, you can build robust, scalable, and cost-effective applications leveraging Azure Blob Storage for storing and accessing your data.
Incorporate these techniques into your workflows to unlock the full potential of Azure Blob Storage in your Python applications.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.