Read Big Azure Blob Storage file – Best practices with examples
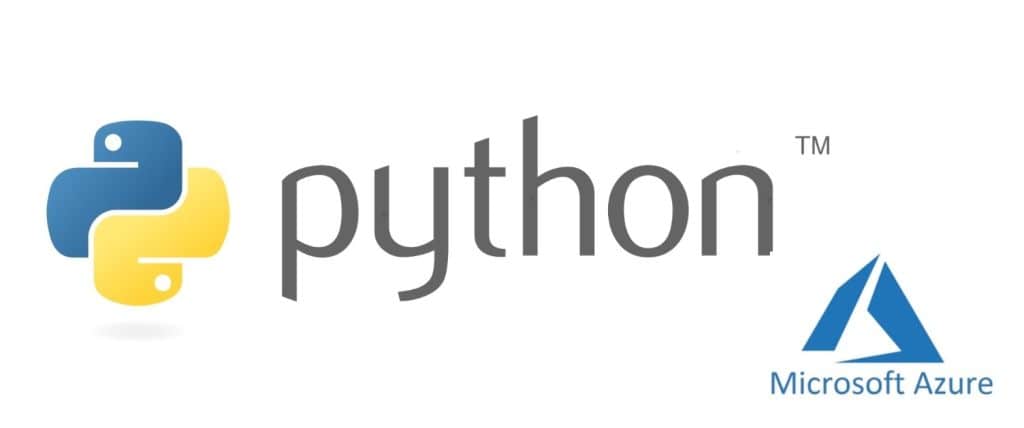
Today in this article, we will see how to Read big Azure blob storage file. Reading a huge file from Azure Blob Storage using Python efficiently involves several best practices to ensure optimal performance, scalability, and reliability.
Today, In this comprehensive guide, we’ll cover various strategies, techniques, and considerations for handling large files in Azure Blob Storage with Python, along with a sample example.
In our last article, we learned how to read basic files from azure blob storage.
Reading a huge file from Azure Blob storage can be done in multiple ways. However, we will cover the technique without downloading the file.
Reading a huge file from Azure Blob Storage in Python without downloading the entire file at once can be achieved using,
- Azure Storage Blob SDK’s
BlobClient
BlobStreamReader
classes.
This approach allows for efficient streaming of the file’s content, reducing memory consumption and improving performance, especially when dealing with large files.
In this detailed explanation, I’ll provide best practices for streaming a large file from Azure Blob Storage in Python, along with a complete example.
Introduction to Azure Blob Storage
Azure Blob Storage is a scalable object storage solution offered by Microsoft Azure, designed to store large amounts of unstructured data such as text or binary data.
It provides features like high availability, durability, and scalability, making it suitable for storing and managing data of any size.
Prerequisites
Before we proceed, ensure you have the following prerequisites:
- An Azure subscription: You’ll need an Azure account to access Azure Blob Storage.
- Azure Storage account: Create a storage account in the Azure portal.
- Azure Storage SDK for Python: Install the
azure-storage-blob
package using pip.
pip install azure-storage-blob
Best Practices for Reading Large Files from Azure Blob Storage
Use Streaming for Large Files
When dealing with large files, it’s essential to use streaming to read data in chunks rather than loading the entire file into memory.
Add the below import statement to your project python file,
from azure.storage.blob import BlobServiceClient from azure.core.exceptions import ResourceNotFoundError
Streaming reduces memory usage and allows for efficient processing of large files without overwhelming system resources.
try:
# Create a blob service client
blob_service_client = BlobServiceClient.from_connection_string(connection_string)
# Get a blob client for the blob
blob_client = blob_service_client.get_blob_client(container=container_name, blob=blob_name)
# Stream the blob's content using BlobStreamReader
with blob_client.get_blob_client() as stream_blob_client:
with stream_blob_client.download_blob() as stream:
# Read the blob's content in chunks
chunk_size = 1024 * 1024 # 1 MB chunk size
offset = 0
while True:
# Read a chunk of data from the stream
chunk = stream.readinto(bytearray(chunk_size))
if not chunk:
break # End of file reached
# Process the chunk (e.g., write to file, perform analysis)
# Example: print the chunk size
print("Read chunk:", len(chunk))
# Update the offset for the next read
offset += len(chunk)
except ResourceNotFoundError as ex:
print("The specified blob does not exist:", ex)
except Exception as ex:
print("An error occurred:", ex)
Example – Please see here a complete example
Optimize the Chunk Size- Azure Blob
To read a huge file from Azure Blob Storage using Python without downloading the entire file at once, you can utilize Azure Blob Storage’s ability to stream data in chunks.
This approach allows you to read the file in smaller pieces, reducing memory usage and improving efficiency, especially for large files.
- Experiment with different chunk sizes to find the optimal balance between network latency, throughput, and memory usage.
- Larger chunk sizes can improve throughput but may increase latency.
- Smaller chunk sizes may reduce latency but result in more overhead.
Here’s an example of how you can achieve this using the azure-storage-blob
library:
Add below import statements to your projects,
from azure.storage.blob import BlobServiceClient from azure.core.exceptions import ResourceNotFoundError
# Define chunk size (in bytes)
chunk_size = 1024 * 1024 # 1 MB chunk size
try:
# Create a blob service client
blob_service_client = BlobServiceClient.from_connection_string(connection_string)
# Get a blob client for the blob
blob_client = blob_service_client.get_blob_client(container=container_name, blob=blob_name)
# Get the blob properties to determine its size
blob_properties = blob_client.get_blob_properties()
# Get the total size of the blob
blob_size = blob_properties.size
# Initialize variables to track the current position and remaining bytes to read
current_position = 0
bytes_remaining = blob_size
# Read the blob in chunks
while bytes_remaining > 0:
# Calculate the chunk size for this iteration
chunk_to_read = min(chunk_size, bytes_remaining)
# Download the chunk of data from the blob
blob_data = blob_client.download_blob(offset=current_position, length=chunk_to_read)
# Process the chunk of data (example: print the chunk)
print("Chunk:", blob_data.readall())
# Update current position and remaining bytes to read
current_position += chunk_to_read
bytes_remaining -= chunk_to_read
except ResourceNotFoundError as ex:
print("The specified blob does not exist:", ex)
except Exception as ex:
print("An error occurred:", ex)
3. Use Parallelism for Concurrent Downloads
To further improve performance, consider downloading chunks of the file in parallel using multiple threads or processes. This approach can leverage the available bandwidth more effectively and reduce overall download time.
4. Handle Retries and Errors Gracefully
Implement retry logic with exponential backoff to handle transient errors such as network timeouts or server failures. Retry policies help ensure robustness and reliability when accessing resources over the network, especially in distributed environments like Azure.
5. Monitor and Log Progress
Include logging and monitoring mechanisms to track the progress of file downloads, detect errors, and troubleshoot performance issues. Logging progress updates and error messages can facilitate debugging and provide visibility into the execution of the download process.
6. Optimize Network Bandwidth
Consider the network bandwidth constraints and optimize the download process accordingly. Techniques like bandwidth throttling, prioritization, and parallelism can help maximize throughput while minimizing network congestion.
7. Use Azure SDK for Python
Utilize the official Azure SDK for Python (azure-storage-blob
) to interact with Azure Blob Storage. The SDK provides high-level abstractions, asynchronous APIs, and built-in features for handling large files efficiently.
Conclusion
Reading large files from Azure Blob Storage with Python requires careful consideration of performance, reliability, and scalability factors. By following best practices such as using streaming, optimizing chunk size, and employing parallelism.
In the provided example, we demonstrated how to download a huge file from Azure Blob Storage in parallel using Python, incorporating these best practices.
By leveraging these techniques, you can effectively manage large-scale data processing tasks and unlock the full potential of Azure Blob Storage for your applications.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.