React – Using Fetch HTTP POST Request Examples

In this article, we shall see how to write React – POST request with easy to understand examples.
Below are the high-level steps which can be performed to be able to use Http services in React application,
- Create a React Component – Function-based and Class-based
- Fetch POST API using State
- Fetch POST API using StateHooks
- Performing POST operation
Before we get started I am assuming you already have a basic understanding of React application.
If not, kindly go through a series of articles on React,
We shall see both approaches like using Function-based Component and Class-based component
Getting Started
Lets create your new React.js Application.
As we saw in our last article Getting started with React , we used a function-based component where we rendered the UI with ‘Hello World’.
Here is below example of class based Component,
Fetch POST example – Using State Object
Let’s add new CustomHttpRequestPost as below. CustomHttpRequestPostHooks is a class-based component and works with Sate objects easily.
import React from 'react';
import logo from './logo.svg';
import './App.css';
class CustomHttpRequestPost extends React.Component {
constructor(props) {
super(props)
this.state = {
date: new Date(),
name: null,
id: null,
}
}
render() {
const { name } = this.state;
const { id } = this.state;
return (
<div className="App">/
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Hello, TheCodeBuzz Community }
</p>
<div className="card-body">
<p>Name: {name}</p>
<p>Id: {id} </p>
</div>
</header>
</div>
);
}
}
export default CustomHttpRequestPost;
In the above example, we shall be updating the State objects like name and id using HTTP GET API response.
We shall be using componentDidMount to call fetch API and we shall re-render the UI with latest values.
Lets define FETCH for POST in componentDidMount a life cycle method,
componentDidMount() {
fetch('https://localhost:5001/api/values', {
method: 'POST', // *GET, POST, PUT, DELETE, etc.
mode: 'cors', // no-cors, *cors, same-origin
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ id: '123', name : 'qweq' })
// body data type must match "Content-Type" header
})
.then(response => response.json())
.then(data => this.setState({ name: data.name, id: data.id }))
}
componentDidMount will get called immediately after component is mounted.
Fetch Post example – Using Hooks
Let’s add new CustomHttpRequestPostHooks component. CustomHttpRequestPostHooks is function based component and works with Hooks.
function CustomHttpRequestPostUsingHooks(){
const [name, setData] = useState(null);
const [id, setId] = useState(null);
useEffect(() => {
fetch('https://localhost:5001/api/values', {
method: 'POST', // *GET, POST, PUT, DELETE, etc.
mode: 'cors', // no-cors, *cors, same-origin
cache: 'no-cache', // *default, no-cache, reload, force-cache, only-if-cached
credentials: 'same-origin', // include, *same-origin, omit
headers: {
'Content-Type': 'application/json'
},
redirect: 'follow', // manual, *follow, error
referrerPolicy: 'no-referrer', // no-referrer, *no-referrer-when-downgrade, origin, origin-when-cross-origin, same-origin, strict-origin, strict-origin-when-cross-origin, unsafe-url
body: JSON.stringify({ id: '123', name : 'qweq' }) // body data type must match "Content-Type" header
})
.then(response => response.json())
.then(data =>
{
setData(data.name);
setId(data.id)
})
},[]);
return (
<div className="App">/
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Hello, TheCodeBuzz Community !
</p>
<div className="card-body">
<p>Name: {name}</p>
<p>Id: {id} </p>
</div>
</header>
</div>
);
}
export default CustomHttpRequestPostUsingHooks;
Above we are making use of useEffect method for putting the Fetch method call as example.
Service POST API returning JSON
Let assume you are getting below HTTP JSON response from Server(Node.js or ASP.NET Core or Spring )
Sample JSON,
{
"id": "d95c1c74-f1c7-480d-8ea1-621327197980",
"name": "TheCodeBuzz"
}
componentDidMount will get called immediately after component is mounted.
Data will be mapped to Fetch result as define in the State or Hooks,

Connecting API/Service endpoint
You are now ready to connect to any REST API endpoint.
I already have below API endpoint which we shall be returning as Employee model (which we have defined as Observable above in getEmployees())
I am using the ASP.NET Core service below. However, as we know REST APIs are language agnostic, you can use Spring, Node.js, Java or Python-based services, etc.
We are connecting to below Service method defined,
[HttpPost]
public ActionResult<Employee> Post([FromBody] Employee value)
{
//Add Employee to Database
listDBEmployee.Add(value);
value.Id = "Post:"+ Guid.NewGuid().ToString();
value.Name = "TheCodebuzz";
return Ok(value);
}
CORS on Server-side
Please note your Server should allow CORS request from your React UI domain.
Please visit for more details: How to Enable CORS in ASP.NET Core REST API
Once the CORS is enabled on your server-side, you shall see the post method hits your API and gives you required response.
Finally , you could see the result in the browser,
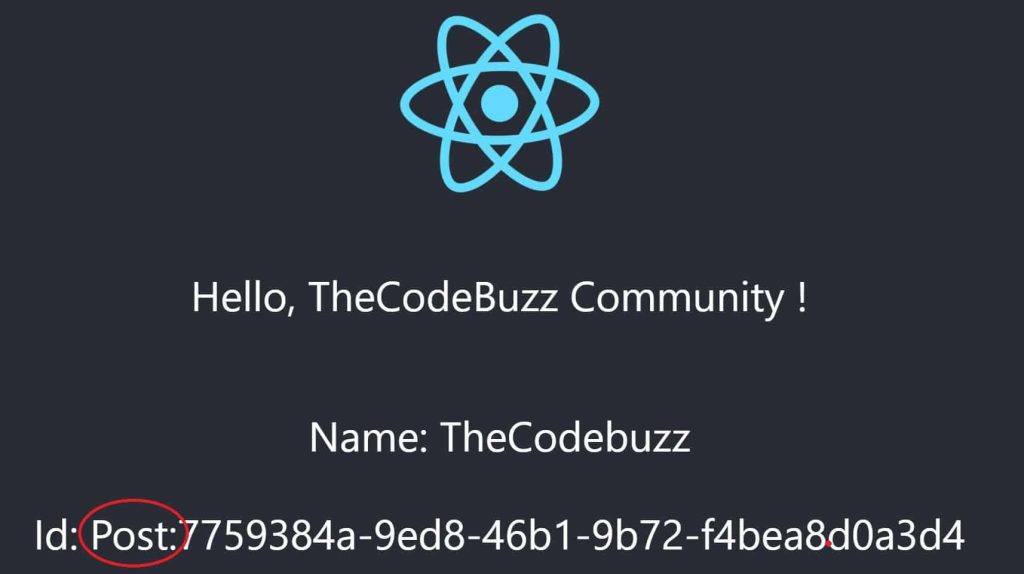
I shall be covering more PUT and Delete methods in my next article.
Kindly stay tuned!!
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
Today in this article we learned how to write simple React– Fetch POST method using State and Hooks using easy to understand examples.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.