Angular Unit Test and Mock HTTPClient GET Request

In this article we will see how to unit test and mock HTTPClient for calls like GET or POST request.
Kindly follow the below steps to set up the Angular testing for the HttpClient request object. We shall follow the Angular Unit Testing Best practices which we covered in our last article.
In unit testing its important to mock the HTTP backend. While writing the Unit test for services using HTTPClient, you may find it is very useful to use HttpClientTestingModule. This module helps in testing and mocking especially data services that make HTTP calls to the servers.
Getting Started
Please import the HttpClientTestingModule and the mocking controller HttpTestingController.
Please add below import statement,
import { HttpTestingController, HttpClientTestingModule } from '@angular/common/http/testing';
Then please add the HttpClientTestingModule to the TestBed.
beforeEach(() => { TestBed.configureTestingModule({ imports: [ HttpClientTestingModule ] });
Example:
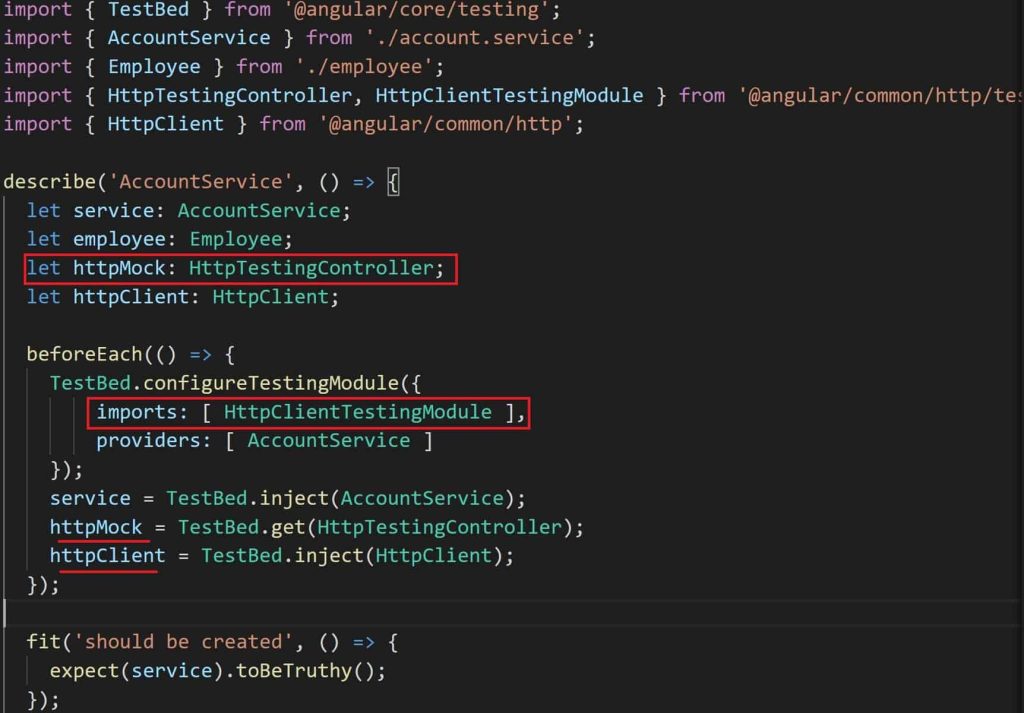
Lets write the test method for GET Http Call.
Target method under test as below.
This method uses Http GET method to get the result over Http.
getEmployees(): Observable<Employee> {
return this.http.get<Employee>(this.apiUrl)
.pipe(
tap(_ => this.log('fetched Employee')),
catchError(this.handleError<Employee>('getEmployees'))
);
}
Below is how TEST method can be written.
it('getEmployees() should call http Get method for the given route', () => {
//Arrange
//Set Up Data
employee = {Name :'TheCodeBuzz', Id: '2131'}
//Act
service.getEmployees().subscribe((emp)=>{
//Assert-1
expect(emp).toEqual(employee);
});
//Assert -2
const req = httpMock.expectOne('/api/values');
//Assert -3
httpMock.verify();
});
Above we have used AAA(Arrange-Act-Assert) pattern to structure our Test cases.
Here is complete Test with additional Asserts,

Above we are performing Multiple Assert which ensures Unit test case pass for all possible scenarios.
Assert 1 – This Verify the observable when it resolves, its result matches test data.
Assert 2– Verify the matched URL get called in the GET API else it throws errors.
Assert 3– Verify that the request called is GET HTTP method only.
Assert 4 – Ensures the correct data was returned using Subscribe callback.
Assert 5 – Ensures that all request are fulfilled and there are no outstanding requests.

Above all measure ensures you writing the robust GET method and you create highly maintainable code.
Using above technique one can write unit test cases for other Http method like POST, DELETE, and PUT method as well.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
References:
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
are any real api calls made to the backend/server when we flush() or expectOne()?
Hello Apoorva- Thanks for your query. Unit Test cases as a good practice should be stateless and should not call over the wire resources. You simply set mock and verify the expected vs actual response for that scenario.
How to test catchError in pipe?
Hey Harry, it’s possible to unit test catch error. I shall soon put an example.