Get Configuration value from appsettings.json in ASP.NET Core – Guidelines

In this article, we will see a few guidelines on how to read Configuration from appsettings.json in ASP.NET Core-based API or MVC application.
If you are interested in loading configuration in .NET Core using dependency injection(DI), you can load key-value pairs via dependency injection using IConfiguration and IOption interface.
Today in this article, we will cover below aspects,
Alternatively, one can use custom configuration instance declaration at the startup and use the configuration across your module including .NET Standards libraries, etc.
So overall in this article, I will talk about the below approaches for reading the configuration.
All below discussed approaches leverage DI and are the best practices dealing with configuration in applications,
- Using IConfiguration
- Using IOptions
- Using Custom Interface
- Using ConfigurationBuilder (non-DI approach)
Getting started
Let’s create MVC or ASP.NET Core API .NET 3.1 or .NET 6 application,

We shall be using below configuration file,
appsettings.json
{
"ServerURL": "https://localhost:44347/api/employee"
"Customer": {
"CustomerKeyurl": "http://thecodebuzz/key",
"CustomerdetailsUrl": "http://thecodebuzz/id",
"Agency": {
"AgencyID": "subvalue1_from_json",
"AccountKey": 200
}
}
}
Please note that CreateDefaultBuilder a Host builder in .NET and ASP.NET Core plays an important role in initializing the Host and its configuration like getting access to applications host details including all the below details,
- Application configuration,
- Logger configuration,
- User secrets,
- Environmental configuration, etc.
It Initializes a new instance of Microsoft.Extensions.Hosting.HostBuilder class with pre-configured defaults.
Approach 1- Using IConfiguration to load the configuration
This is the simplest way of loading configuration. This doesn’t require you to write any custom interface etc.
Please note that when you define your host with CreateDefaultBuilder l It loads Application Configuration defined ASP.NET Core appsettings.json in ASP.NET Core.
This application configuration is available to you out of the box and accessible through the IConfiguration interface by default.
If you are using a non-prod environment like DEV or TEST then such environment-specific configuration details will also be available from appsettings.[EnvironmentName].json
By default, the IConfiguration interface instance will load everything and let you retrieve the required key-value pair-specific config details.
Example:
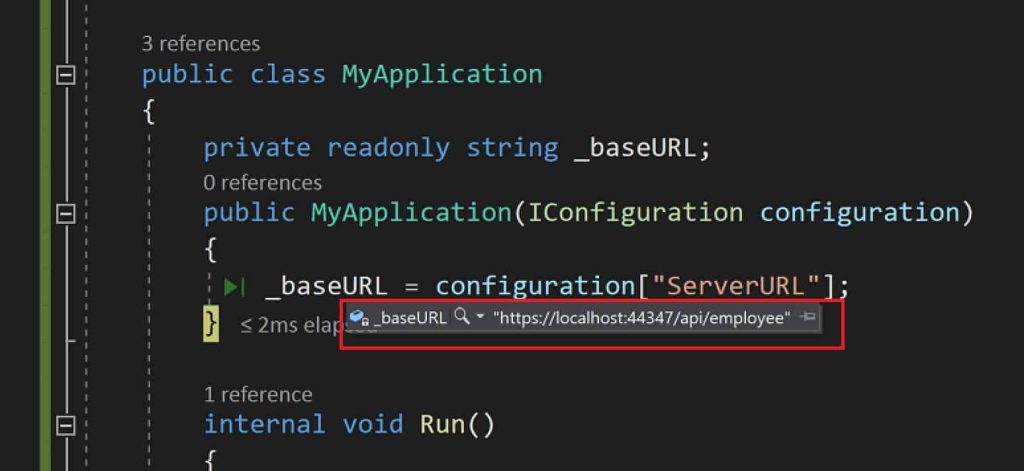
Approach 2- Using IOptions load the configuration
Using IOptions interface pattern gives access to configuration details in related classes and has the choice to load config data based on the need.
Here we will be using IOptions interface to load the configuration as needed in the application.
Example
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddSingleton<IUniqueIdService, UniqueIdService>();
services.Configure<CustomerConfig>(Configuration.GetSection("Customer"));
..
..
}
Above using IOptions interface load the required configuration easily as shown below,
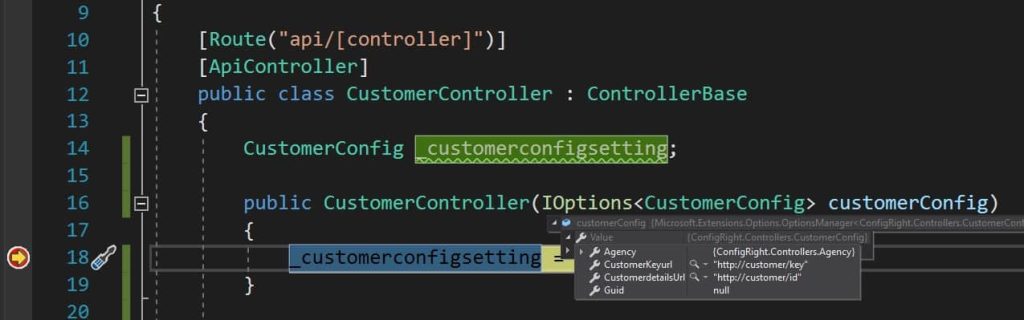
CustomerConfig class is the mapping class created using the JSON schema defined in the apsettings.json file.
The same techniques are discussed in the articles on Creating Typesafe Configuration in ASP.NET Core.
Approach 3 – Using a Custom interface to load the configuration details
In this approach, you write your custom interface to load the specific configuration details within the ConfigureServices method and resolve the interface.
Example:

In the above example, you can use the ICustomerConfig interface as your configuration object defined within Startup.cs file.
Once defined depending upon the lifetime management the instance will help you to define DI the instance in any other module in your application.
For more details on this approach please visit the below article.
Approach 4 – Loading configuration without Dependency injection i.e ConfigurationBuilder
Load any configuration like apsettings.json or .ini or XML or app.config, etc without dependency injection(DI).
Please see the below article for complete details.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Additional references
That’s All,
Happy Coding!
Summary
In this article, we looked at how to load/read configuration from the appsettings.json file in the .NET core-based application like API or MVC leveraging dependency injection(DI). We looked at how easily one can read the configuration Using IConfiguration or Using IOptions or can write Custom Interface as well to perform the same task.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.