Load Configuration in .NET Core Console or WinForms application- Part II

Today in this article we shall cover , How to Load Configuration in .NET Core Console or WinForms application.You can load configuration details from different file configuration providers like JSON, INI or XML files in .NET Core Windows or WPF applications easily.
Today in this article, we will cover below aspects,
I already covered the loading of Apsetting.json or Custom JSON file loading in the below article in detail,
This loading is similar to ASP.NET Core-based configuration loading which we learned in the previous article but there is a difference on how we instantiate Hostbuilder for a non-host application like Console or WPF or Windows application.
The below-discussed techniques actually work fine for all non-host applications like Windows Form or WPF or Console applications using Generic Host Builder for building IoC containers.
- Application Configuration File(apsettings.json)
- Loading (.INI) File configuration
- Loading (XML) File configuration
- Loading any Custom(JSON)
- Loading configuration without Dependency injection
Loading Apsettings.json file
Discussed already in details – Application Configuration File(apsettings.json)
Loading INI File in Console application
To load INI file.NET Core provides INI Configuration Provider which loads configuration from INI file(key-value pairs) at runtime.
Please install Nuget package in Console or Win Form or WPF app,
PM> Install-Package Microsoft.Extensions.Configuration.Ini -Version 3.1.2
Please call AddIniFile extension method on an instance of ConfigurationBuilder as shown below, ,
var hostBuilder = Host.CreateDefaultBuilder()
.ConfigureAppConfiguration((context, builder) =>
{
// Add ini configuration files...
builder.AddIniFile("Config.ini", optional: true);
})
.ConfigureServices((hostContext, services) =>
{
services.AddTransient<MyApplication>();
}).UseConsoleLifetime();
var builderDafault = hostBuilder.Build();
...
...
Here is a sample .ini file is as below,
[section0]
key0=value
key1=value
[section1]
subsection:key=value
[section2:subsection0]
key=value
[section2:subsection1]
key=value
Let’s execute the application and verify the configuration details,
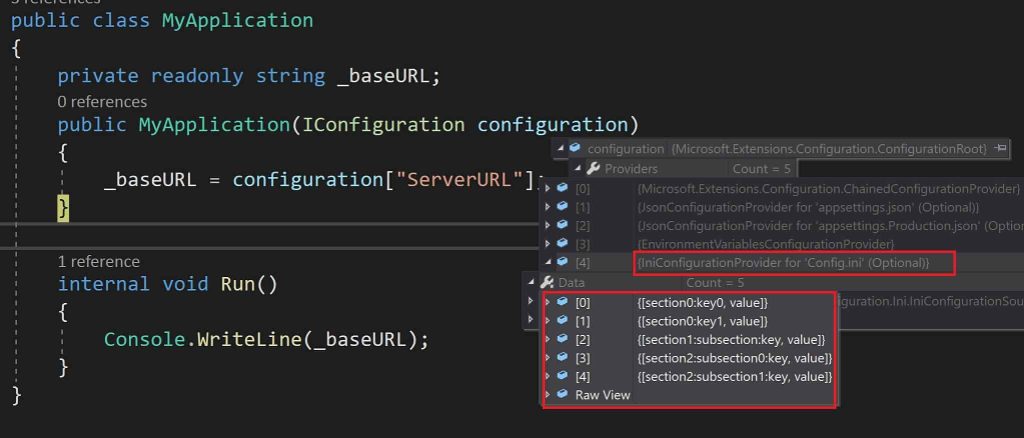
Loading XML File in Console application
If you want to load an XML file please XML Configuration Provider.
Please install below Nuget package,
PM> Install-Package Microsoft.Extensions.Configuration.Xml -Version 3.1.2
To use XML configuration at the runtime, Please call AddXmlFile extension method on an instance of ConfigurationBuilder as shown below,
var hostBuilder = Host.CreateDefaultBuilder()
.ConfigureAppConfiguration((context, builder) =>
{
// laod XML files...
builder.AddXmlFile("Config.xml", optional: true);
})
.ConfigureServices((hostContext, services) =>
{
services.AddTransient<MyApplication>();
}).UseConsoleLifetime();
var builderDafault = hostBuilder.Build();
...
...
Sample custom XML file as below,
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<DataBase>
<Name>TEST</Name>
<key1>"Server=localhsot\\sql;database=master;"</key1>
</DataBase>
<Application>
<key0>value</key0>
<key1>value</key1>
</Application>
</configuration>
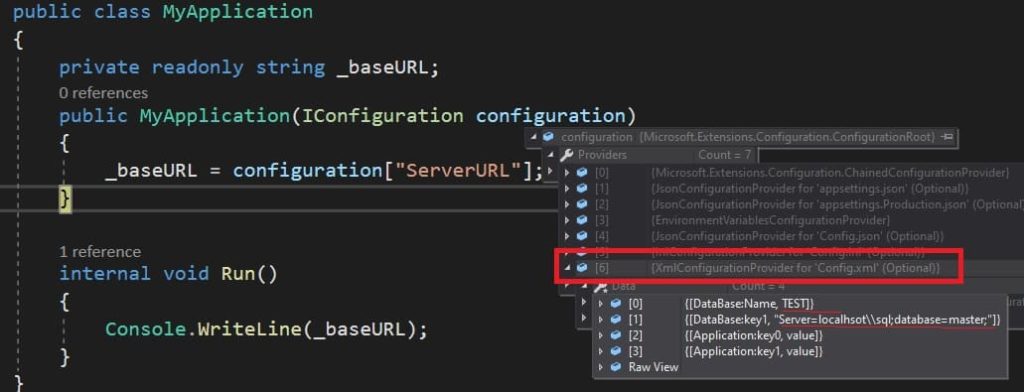
In all of the above discussed FileConfigurationProvider, related the extension methods like AddJsonfile() or AddIniFile() or AddXmlFile lets you specify parameters like If the file is optional or not, also if the option to specify reload is required or not.
Strongly Typed configuration
One can generate strongly typed configuration as discussed in the article below,
References: Configuration in ASP.NET Core
Loading configuration without Dependency injection i.e using ConfigurationBuilder
Load any configuration like apsettings.json or .ini or XML or app.config, etc without dependency injection(DI). Please see the below article for complete details.
That’s All. Happy Coding!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
Thanks much. Very helpful
Thanks Ajay for the feedback!