React – Passing Data From Child Component To Parent Component communication
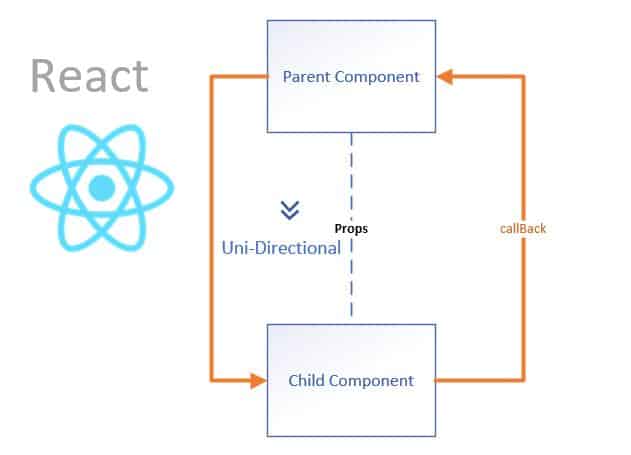
Today in this article we shall learn how to perform component interactions in the react application where we shall see React – Child To Parent Component communication and passing data from the Parent component to the Child Component.
In our last article on Passing Data From Parent to Child Component, we already learned how to pass data using the Props system from a Parent Component to Child Component easily.
We shall cover below basic aspects in today’s article,
- Getting Started
- Create Parent Component – LoginUserComponent
- React Create Child Component – SelectMovieComponent
- Update Parent State object with Child data
- What is Redux and what use cases around its usage?
- I shall be covering Redux usage and when to use it and when not in my next article! Until then please stay tuned!
- Summary
Before we get started, I am assuming you already have a basic understanding of React applications.
If not, kindly go through a series of articles on React,
Getting Started
Let’s get started step by step to perform data transfer from the Child to the Parent component

So initially we shall be focussing on how we provide information from the Child to the Parent.
Then In the second stage, we shall see how to consume data from the Parent components.
I shall be using very basic two components defined as below
- Parent Component – LoginUserComponent
- Child Component – SelectMovieComponent
Create Parent Component – LoginUserComponent
Let’s create a simple Parent Component – UserComponent as below,
class LoginUserComponent extends React.Component {
onSearchSubmit(movie) {
console.log('Input received in Parent Component');
console.log(movie);
}
render() {
return (
<div className="ui container">
<SelectMovieComponent onSubmit= { this.onSearchSubmit } />
</div>
);
}
}
export default LoginUserComponent;
Above we have used the SelectMovieComponent using a naming pattern as we discussed in our last article on Props system,
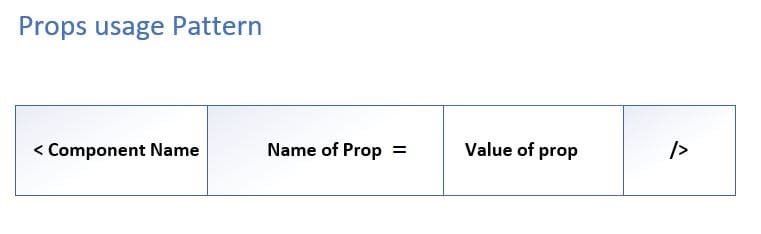
React Create Child Component – SelectMovieComponent
Similarly, Let’s create a simple Child Component – SelectMovieComponent as below,
class SelectMovieComponent extends React.Component {
state = { movieSelected: '' };
onMoveSelected = (event) => {
event.preventDefault();
this.props.onSubmit(this.state.movieSelected);
console.log(this.state.movieSelected);
}
render() {
return (
<div className="ui segment">
<form onSubmit={this.onMoveSelected} className="ui form">
<div className="field">
<label>Movie Search</label>
<input
type="text"
onChange={e => this.setState({ movieSelected: e.target.value })}
value={this.state.movieSelected}
/>
<div>
Movie selected by User: {this.state.movieSelected}
</div>
</div>
</form>
</div>
);
}
}
export default SelectMovieComponent;
Above in Child Component – SelectMovieComponent we performed the following,
- Create a Class-Based component SelectMovieComponent
- Create a State object movieSelected
- Update State object movieSelected in the OnChange event with the current value
- Submit the Form by calling onMovieSelected
- Update the props with the same name as indicated in the Parent i.e using this.props.onSubmit(this.state.movieSelected);
Once you launch the app, you shall see both the components execute and produces the below result on the UI,

Above we console printed the result from the Child into the Parent component.
Let’s now enhance and use the data in Parent as a state object.
Update Parent State object with Child data
Please update the Parent code to receive Child data into the state object of the Parent component as below,
class LoginUserComponent extends React.Component {
state = { movieReceived: '' };
onSearchSubmit(movie) {
console.log('Input received in Parent Component');
console.log(movie);
this.setState({movieReceived:movie})
}
render() {
return (
<div className="ui segment">
<div className="ui container">
<SelectMovieComponent onSubmit={this.onSearchSubmit.bind(this)} />
</div>
<div>
Movie received by User(parent): {this.state.movieReceived}
</div>
</div>
);
}
}
Kindly note call-back method is updated with the bind(this) object.
Once you execute you shall see the result in the browser as below,

That’s All!
What is Redux and what use cases around its usage?
Once we go beyond and master component communication, you might need to think of proper state management in your application (especially if dealing with large enterprise applications).
I shall be covering Redux usage and when to use it and when not in my next article! Until then please stay tuned!
References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
Today in this article we understood further Component communication where we performed Child to Parent communication in React programming. We understood the props system and understood the naming pattern and also used the State object and callback event for passing data from Child to Parent easily.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.