Send HTTP POST request in .NET

In today’s post, we will write client-side code to Send HTTP POST requests in .NET.
We will see the technique of regular HTTPClient and also use HttpClientFactory (recommended) examples
Let’s look at a few examples to invoke HTTP POST methods using HttpClientfactory.
As discussed in the previous article I have already setup the named HttpClientclientFactory to create the required HttpClient instance as below,
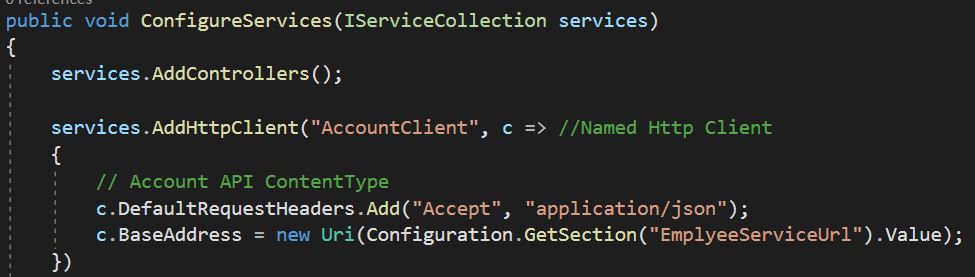
Send HTTP POST request in .NET
Below is a sample POST method example.
Below we are creating an instance of HttpClient using HttpClientFactory and invoking the POST method asynchronously.
One can create a HttpClient instance using a static instance or named or typed instances as needed.
Note: Below samples are shown using direct usage of Post call in Controller.
Ideally please structure your code considering the separation of concerns using Repository pattern etc.
Ideally, the POST method should also return the location of newly created resources as per REST guidelines.
[HttpPost]
public async Task<IActionResult> Post([FromBody]Customer customer)
{
HttpContent httpContent = new StringContent(JsonConvert.SerializeObject(customer)
, Encoding.UTF8, "application/json");
HttpResponseMessage response = await client.PostAsync("api/acounts", httpContent);
if (response.IsSuccessStatusCode)
{
return Ok(response.Content.ReadAsStreamAsync().Result);
}
else
{
return StatusCode(500, "Something Went Wrong! Error Occured");
}
}
The above-discussed HttpClient object can be created using any of the below methods mentioned and can be initialized in the Controller constructor,
HttpClientfactory alternative ?
If you are not using the HttpClientfactory instance then please see below the way to initialize the HttpClient instance,
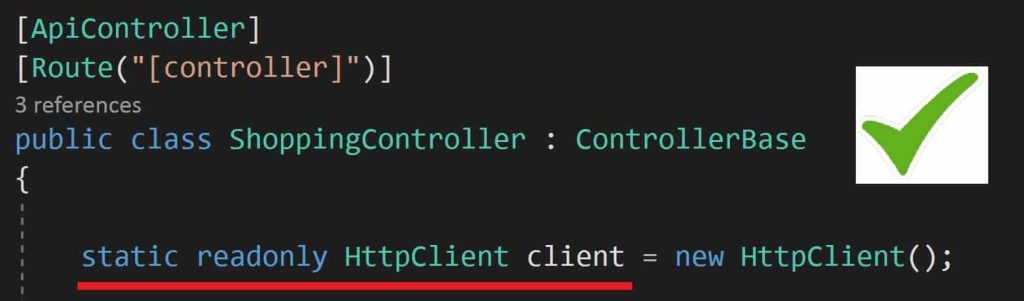
References:
That’s all! Happy coding!
Does this help you fix your issue?
Do you have any better solutions or suggestions? Please sound off your comments below.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.