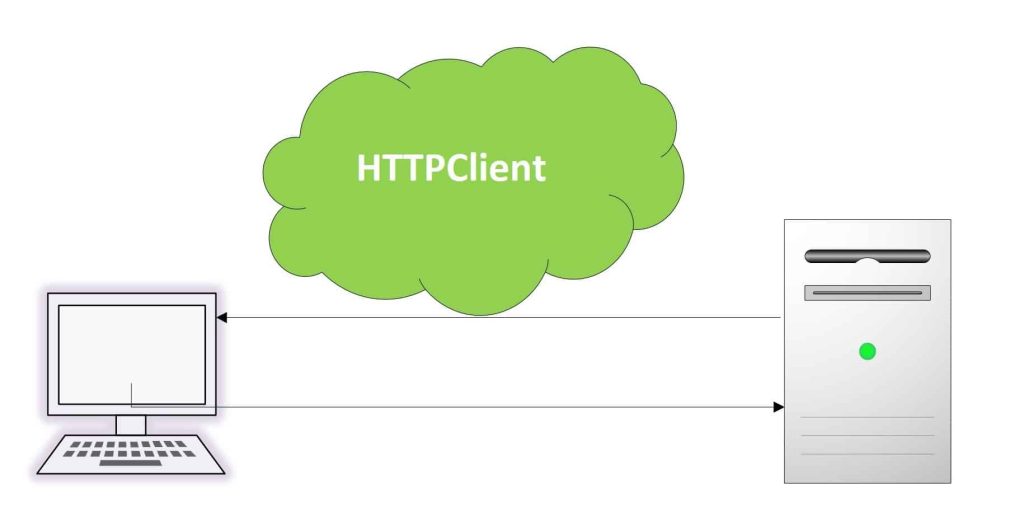
Today in this article, we will see how to use and leverage ProblemDetails in ASP.NET Core API or MVC application.
In our last article on RFC7807 Problem Details guidelines, we understood how to represent HTTP status codes and associated details.
However, Until the RFC7807 specification was released in 2016, there was no uniform format for the HTTP Error response representation.
Today in this article, we will cover below aspect,
Let’s take a simple example below where we shall use standard HTTP status code returning it from the Controller method.
[HttpGet]
[Route("Book")]
public async Task<string> GetAsyncProblemDetails(string id)
{
Book book;
try
{
if(string.IsNullOrEmpty(id))
{
return BadRequest("Invalid Input");
}
//await method - Asynchronously
book = await _bookService.GetBookAsync(id);
if (book == null)
{
return NotFound("Books not found");
}
}
catch (Exception ex)
{
_logger.LogError(ex.Message);
}
// do more stuff
return Ok(book);
}
In the above code, we are returning standards HTTP-supported classes provided by the .NET framework
Example
- NotFound() or
- Ok()
- BadRequest()
Below is the class response with the HTTP status code by the client.
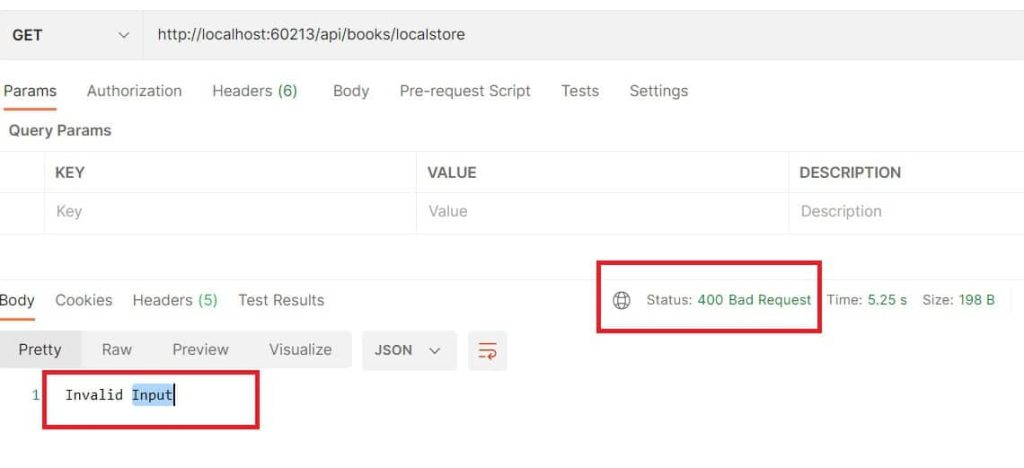
Let now implement the ProblemDetails for the same above HTTP Status code with more details,
[HttpGet]
[Route("Book")]
public async Task<IActionResult> GetAsync(string id)
{
Book book = null;
try
{
if (string.IsNullOrEmpty(id))
{
return BadRequest(new ProblemDetails
{
Title = "Invalid Books",
Detail = $"Book id is invalid. Books id with null or empty strings
not allowed",
Type = "https://www.thecodebuzz.com/get-free-ebook-micro-services-
guidelines/probl/book-invalid",
Instance = HttpContext.Request.Path,
});
}
Using problem details structure, below is how the Response object will be constructed,

The above-used attribute for the ProblemDetails class is defined below. The below attributes are based on the RFC
Where,
- type : A URI reference that identifies the problem type and provides human-readable documentation for the problem type.
- title : It provides documentation for the problem type in a human-readable format.
- status : For this occurrence of the problem, the origin server generated an HTTP status code.
- details : A human-readable explanation of the problem instance, explaining problem details in this specific case.
- instance : URI reference that identifies the problem instance. It may or may not yield further information if dereferenced.
Controller method returning IActionResult
If your controller method already uses IActionResult as the return type for any action or HTTP operations then the ProblemDetails class is already built into it as the default RFC implementation.
All you need is the controller returning IActionResult for the method as explained below,
References :
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.